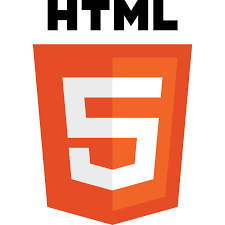
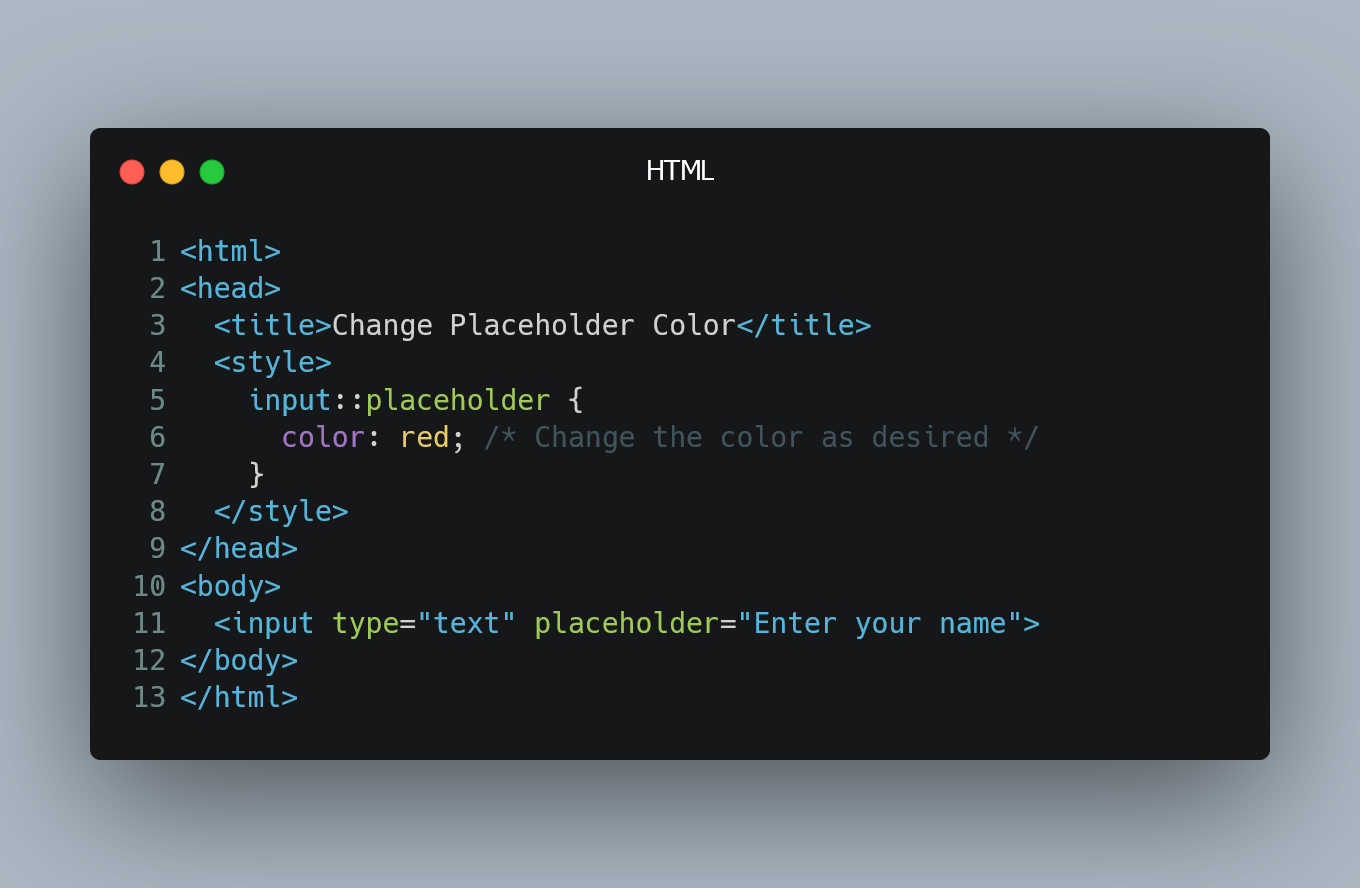
You can easily change the color of an HTML input's placeholder text using CSS. The placeholder text is the text that appears inside an input field as a hint for the user about the expected input.
#1. Basic CSS Styling
To change the color of the placeholder text, target the ::placeholder
pseudo-element in your CSS.
<!DOCTYPE html>
<html>
<head>
<title>Change Placeholder Color</title>
<style>
/* Target the placeholder pseudo-element */
input::placeholder {
color: red; /* Change the color as desired */
}
</style>
</head>
<body>
<input type="text" placeholder="Enter your name">
</body>
</html>
In this example, we use the input::placeholder
selector to target the placeholder pseudo-element of the input element. We then set the color
property to the desired color, in this case, red
.
#2. Using RGBA or HEX Colors
You can use different color formats, such as RGBA or HEX, to style the placeholder text.
<!DOCTYPE html>
<html>
<head>
<title>Change Placeholder Color</title>
<style>
/* Using RGBA */
input::placeholder {
color: rgba(0, 128, 0, 0.8); /* Green with 0.8 opacity */
}
/* Using HEX */
input::placeholder {
color: #ff6600; /* Orange color */
}
</style>
</head>
<body>
<input type="text" placeholder="Enter your email">
</body>
</html>
In these examples, we use RGBA and HEX color formats to set the color of the placeholder text to green with 80% opacity and orange, respectively.
#3. Using Vendor Prefixes for Browser Compatibility
For maximum browser compatibility, consider using vendor prefixes for the ::placeholder
pseudo-element.
<!DOCTYPE html>
<html>
<head>
<title>Change Placeholder Color</title>
<style>
/* Vendor prefixes for browser compatibility */
input::-webkit-input-placeholder {
color: blue; /* Blue color for WebKit-based browsers */
}
input::-moz-placeholder {
color: blue; /* Blue color for Firefox */
opacity: 1; /* Opacity is required for Firefox */
}
input:-ms-input-placeholder {
color: blue; /* Blue color for Microsoft Edge */
}
input::placeholder {
color: blue; /* Default color for other modern browsers */
}
</style>
</head>
<body>
<input type="text" placeholder="Enter your password">
</body>
</html>
In this example, we provide vendor-prefixed versions of the ::placeholder
pseudo-element for WebKit-based browsers (e.g., Chrome, Safari), Firefox, and Microsoft Edge. The final input::placeholder
rule sets the default color for other modern browsers.
0 Comment