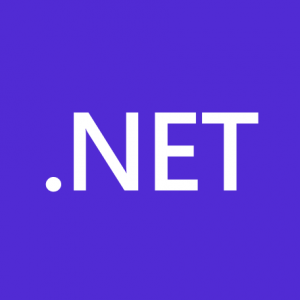
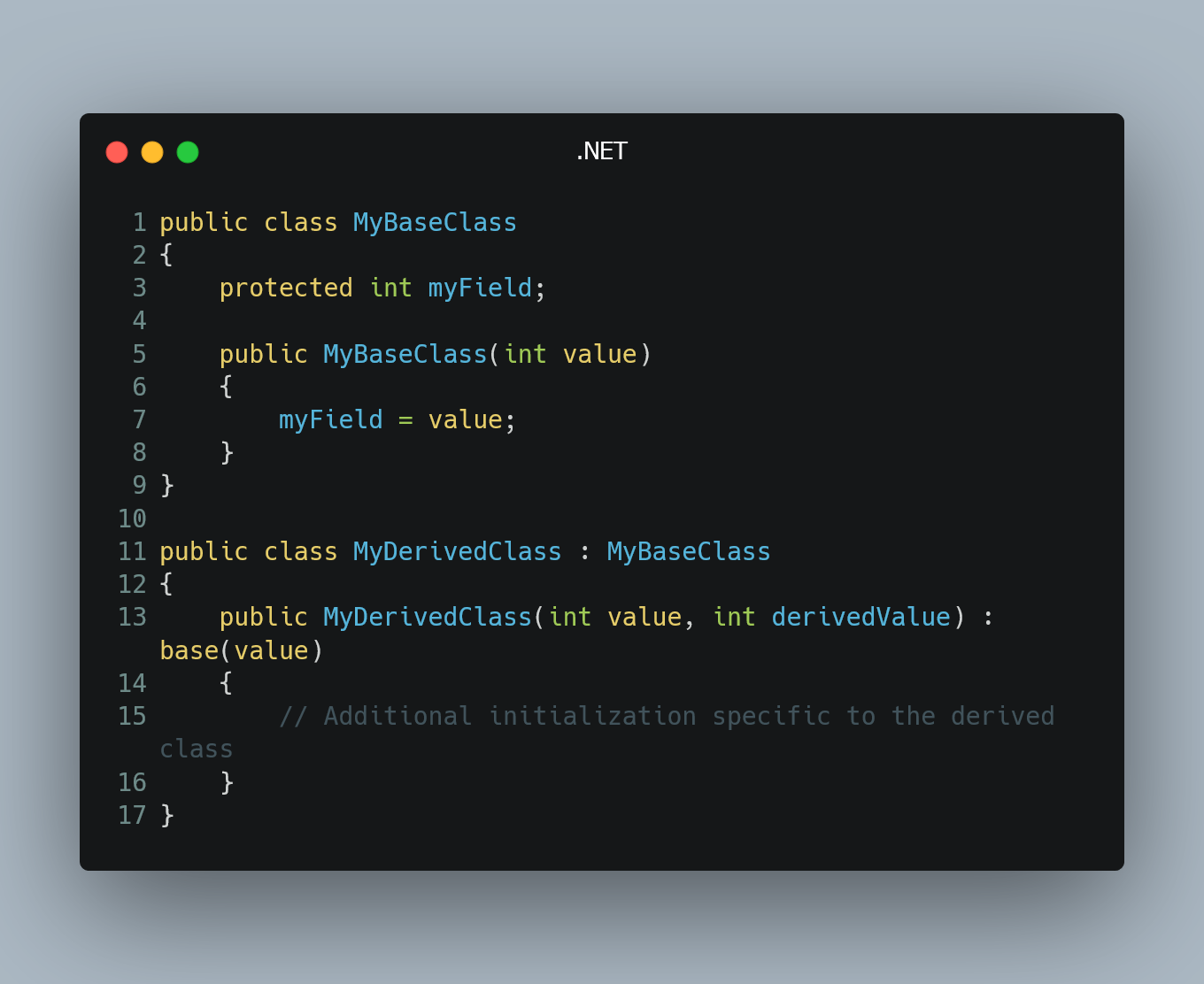
In C#, when you create a derived class (also known as a subclass), it can inherit members from its base class (also known as a superclass).
The Need for Base Constructors
Before diving into calling the base constructor, let's understand why it is essential. The base constructor is responsible for initializing the inherited members from the base class. If the base class has required parameters in its constructor, you must call the base constructor to ensure that the derived class is correctly initialized.
Calling the Base Constructor Syntax
To call the base constructor in the derived class, use the base
keyword followed by parentheses with any required arguments.
Example:
public class MyBaseClass
{
protected int myField;
public MyBaseClass(int value)
{
myField = value;
}
}
public class MyDerivedClass : MyBaseClass
{
public MyDerivedClass(int value, int derivedValue) : base(value)
{
// Additional initialization specific to the derived class
}
}
In this example, MyDerivedClass
inherits from MyBaseClass
. The constructor of MyDerivedClass
takes two parameters, one for the base class and one specific to the derived class. By using base(value)
in the derived class constructor, we call the base constructor with the provided value, ensuring the myField
member in the base class is initialized.
Calling the Default Base Constructor
If the base class has a parameterless constructor (default constructor), you don't need to explicitly call it. The base constructor is automatically called when creating an object of the derived class.
Example:
public class MyBaseClass
{
public MyBaseClass()
{
// Parameterless constructor
}
}
public class MyDerivedClass : MyBaseClass
{
// No need to call the base constructor explicitly
}
In this example, MyDerivedClass
inherits from MyBaseClass
, and since MyBaseClass
has a default constructor, it is automatically invoked when creating an instance of MyDerivedClass
.
0 Comment