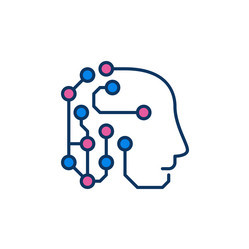
Calculating Distance Between Two Latitude-Longitude Points using the Haversine Formula
The Haversine formula is a mathematical formula used to calculate the distance between two points on the surface of a sphere, such as the Earth. When dealing with latitude-longitude points, this formula can be employed to determine the straight-line distance (as the crow flies) between two geographical locations.
Understanding the Haversine Formula
What is the Haversine Formula?
The Haversine formula is based on trigonometric functions and uses the latitude and longitude of two points to compute the distance between them. It assumes the Earth as a perfect sphere, which makes it suitable for relatively short distances.
Formula Equation
The Haversine formula is represented as:
a = sin²(Δφ/2) + cos(φ1) * cos(φ2) * sin²(Δλ/2)
c = 2 * atan2(√a, √(1-a))
d = R * c
Where:
- φ1 and φ2 are the latitudes of the two points in radians.
- Δφ is the difference in latitude between the two points in radians.
- Δλ is the difference in longitude between the two points in radians.
- R is the radius of the Earth (mean radius = 6,371 km).
Implementing the Haversine Formula
Using JavaScript
function haversineDistance(lat1, lon1, lat2, lon2) {
const R = 6371; // Earth's radius in km
const dLat = (lat2 - lat1) * Math.PI / 180;
const dLon = (lon2 - lon1) * Math.PI / 180;
const a =
Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(lat1 * Math.PI / 180) * Math.cos(lat2 * Math.PI / 180) *
Math.sin(dLon / 2) * Math.sin(dLon / 2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
const distance = R * c;
return distance;
}
// Example usage:
const distance = haversineDistance(52.5200, 13.4050, 48.8566, 2.3522);
console.log(distance); // Output: 878.48 km
Using Python
import math
def haversine_distance(lat1, lon1, lat2, lon2):
R = 6371.0 # Earth's radius in km
d_lat = math.radians(lat2 - lat1)
d_lon = math.radians(lon2 - lon1)
a = math.sin(d_lat / 2) * math.sin(d_lat / 2) + math.cos(math.radians(lat1)) * math.cos(math.radians(lat2)) * math.sin(d_lon / 2) * math.sin(d_lon / 2)
c = 2 * math.atan2(math.sqrt(a), math.sqrt(1 - a))
distance = R * c
return distance
# Example usage:
distance = haversine_distance(52.5200, 13.4050, 48.8566, 2.3522)
print(distance) # Output: 878.48 km
In both JavaScript and Python examples, the Haversine formula is implemented to calculate the distance between two latitude-longitude points. The result is the distance in kilometers (km) as the crow flies between the two locations.
0 Comment