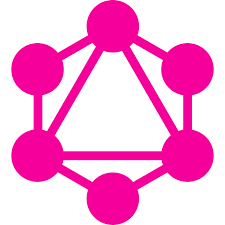
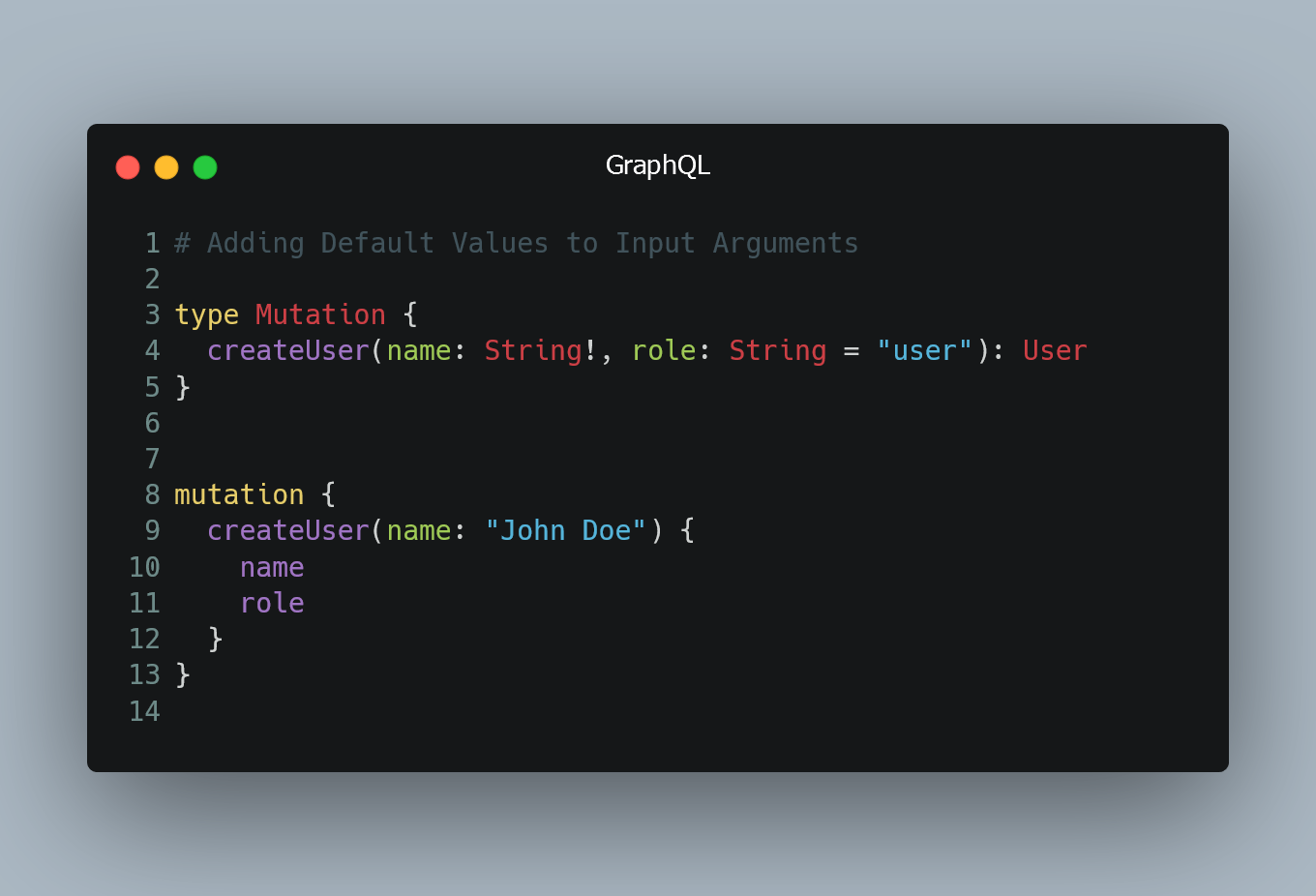
In GraphQL, you can set default values for input arguments, ensuring that certain fields or arguments have predefined values if not explicitly provided by the client. This feature is useful when you want to simplify queries or mutations by automatically filling in some arguments with common or fallback values.
Defining Default Values in the Schema
To add default values to input arguments, you need to define the default value directly in the GraphQL schema. This is done by adding the default value as part of the argument definition.
Consider the following example, where we have a simple mutation to create a new user. We want to set the role
argument to a default value of "user" if the client doesn't explicitly provide it.
type Mutation {
createUser(name: String!, role: String = "user"): User
}
In the above schema, the role
argument has a default value of "user".
Utilizing the Default Values
When the client sends a mutation without providing a value for the role
argument, the GraphQL server will automatically use the default value "user".
Example Mutation Request:
mutation {
createUser(name: "John Doe") {
name
role
}
}
Corresponding Request Sent to the Server:
mutation {
createUser(name: "John Doe", role: "user") {
name
role
}
}
As shown in the example, since the client did not provide a value for the role
argument, the GraphQL server used the default value "user" for the role
field in the response.
Default Values for Input Object Types
You can also apply default values to fields within input object types. For example:
input CreateUserInput {
name: String!
role: String = "user"
}
type Mutation {
createUser(input: CreateUserInput!): User
}
Here, the role
field within the CreateUserInput
input object type has a default value of "user".
Caution with Nullable Fields
When setting default values for input arguments or fields, be cautious when using nullable types. If a default value is defined for a nullable type, it will be used when the client explicitly provides null
as the argument value.
0 Comment