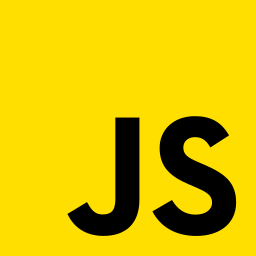
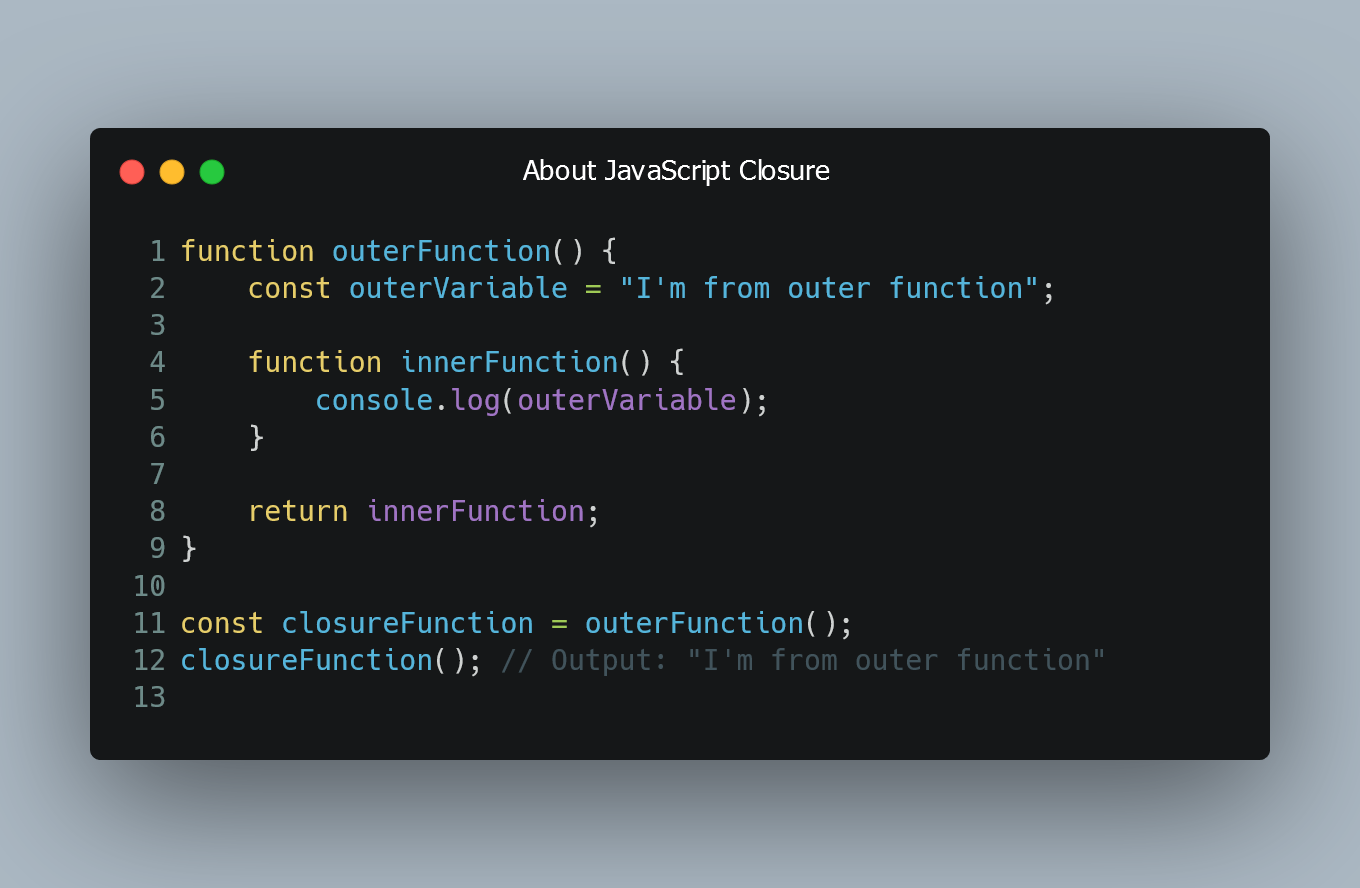
In JavaScript, closures are an important and powerful concept that enables functions to remember the environment in which they were created.
What is a Closure?
A closure is a function that retains access to its own scope, even after the function has finished executing. It "closes over" the variables from its outer (enclosing) function scope, allowing those variables to persist beyond the lifetime of the function itself.
Creating a Closure
Closures are created when a function is defined within another function and then returned or passed as an argument to other functions.
Example
function outerFunction() {
const outerVariable = "I'm from outer function";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Output: "I'm from outer function"
In this example, innerFunction
is a closure because it is defined within outerFunction
and still has access to the outerVariable
even after outerFunction
has finished executing.
Preserving State with Closures
Closures are useful for preserving state in JavaScript. Since closures remember their lexical environment, they can be used to store data across multiple function calls.
Example
function counter() {
let count = 0;
function increment() {
count++;
console.log(count);
}
return increment;
}
const counterFunction = counter();
counterFunction(); // Output: 1
counterFunction(); // Output: 2
counterFunction(); // Output: 3
In this example, counter
creates a closure increment
that maintains its own count
variable, allowing the increment
function to remember the state between calls.
Private Variables with Closures
Closures are also useful for creating private variables in JavaScript.
Example
function createPerson(name) {
return function() {
console.log("Hello, my name is", name);
};
}
const person1 = createPerson("Alice");
const person2 = createPerson("Bob");
person1(); // Output: "Hello, my name is Alice"
person2(); // Output: "Hello, my name is Bob"
In this example, createPerson
returns a closure that captures the name
variable, effectively creating private variables for each person.
0 Comment