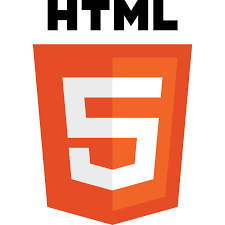
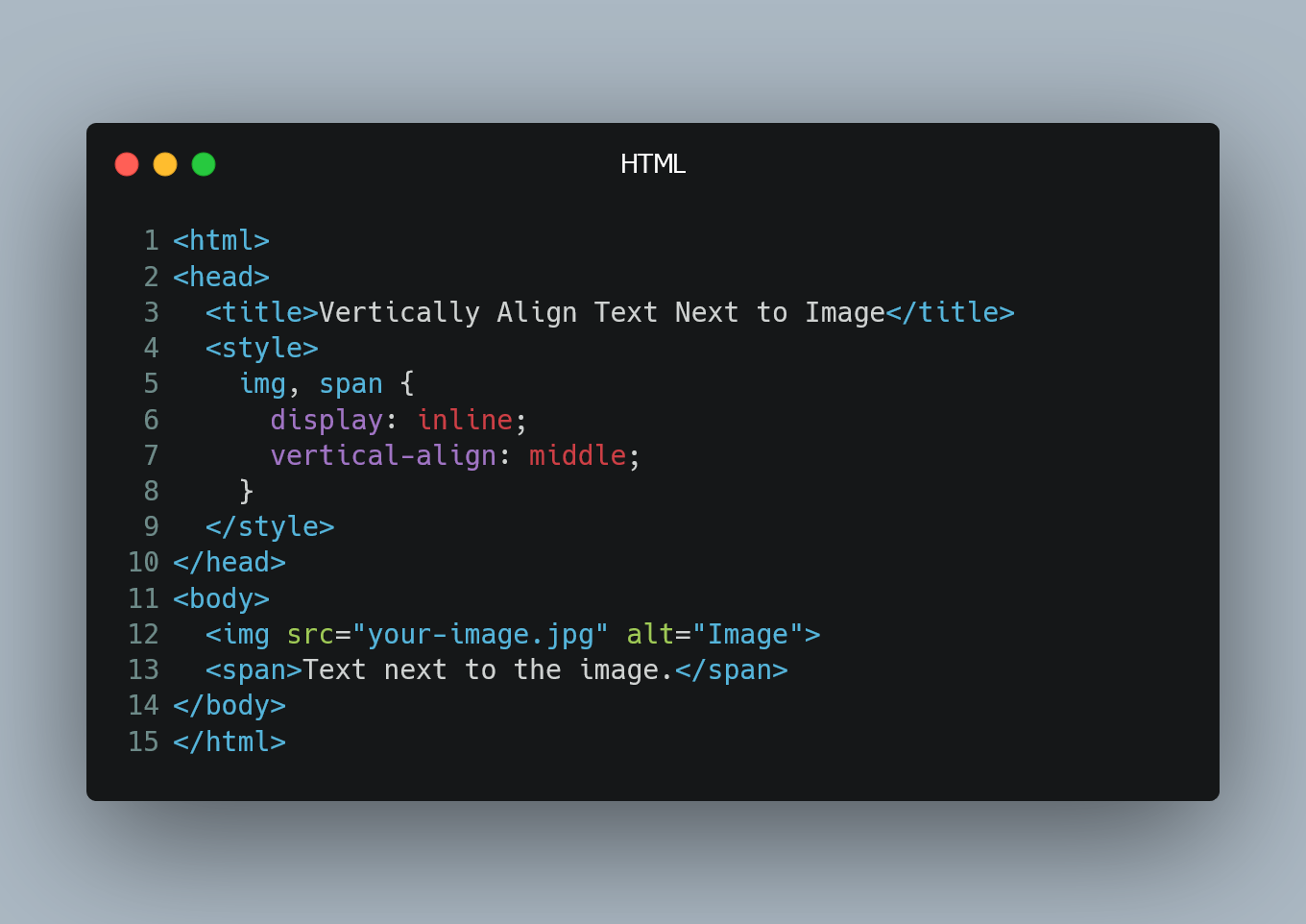
To vertically align text next to an image in HTML and CSS, you can use the vertical-align
property along with the appropriate display and positioning properties.
#1. Using "vertical-align" with Inline Elements
If your image and text are both inline elements (e.g., <img>
and <span>
), you can use the vertical-align
property to align them vertically.
<!DOCTYPE html>
<html>
<head>
<title>Vertically Align Text Next to Image</title>
<style>
/* Set the image and text to inline elements */
img, span {
display: inline;
vertical-align: middle;
}
</style>
</head>
<body>
<img src="your-image.jpg" alt="Image">
<span>Text next to the image.</span>
</body>
</html>
In this example, we set the <img>
and <span>
elements to display: inline;
, which allows them to be aligned horizontally in the same line. The vertical-align: middle;
property is then applied to both elements to vertically center the text next to the image.
#2. Using "vertical-align" with Block-Level Elements
If your image or text is a block-level element (e.g., <div>
), you can use a combination of display: inline-block;
and vertical-align
to achieve vertical alignment.
<!DOCTYPE html>
<html>
<head>
<title>Vertically Align Text Next to Image</title>
<style>
/* Set the image to inline-block and vertical-align middle */
img {
display: inline-block;
vertical-align: middle;
}
/* Set the text to display as inline-block and vertical-align middle */
.text {
display: inline-block;
vertical-align: middle;
}
</style>
</head>
<body>
<img src="your-image.jpg" alt="Image">
<div class="text">Text next to the image.</div>
</body>
</html>
In this example, the <img>
element is set to display: inline-block;
and vertical-align: middle;
, while the text is wrapped inside a <div>
with the class .text
, which is also set to display: inline-block;
and vertical-align: middle;
. This ensures that both the image and text are aligned vertically in the middle.
#3. Using Flexbox
Another modern approach is to use Flexbox, which provides a more flexible and powerful way to align elements.
<!DOCTYPE html>
<html>
<head>
<title>Vertically Align Text Next to Image</title>
<style>
/* Create a flex container */
.flex-container {
display: flex;
align-items: center;
}
</style>
</head>
<body>
<div class="flex-container">
<img src="your-image.jpg" alt="Image">
<span>Text next to the image.</span>
</div>
</body>
</html>
In this example, we create a <div>
with the class .flex-container
and set it as a Flexbox container using display: flex;
. The align-items: center;
property aligns the items (the image and text) vertically in the center of the container.
0 Comment