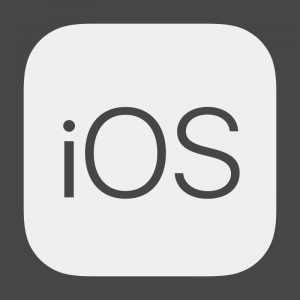
Published in
iOS
Interval matching with switch statements in Swift allows you to handle cases where the value falls within specific ranges or intervals.
Interval Matching with Switch
With Swift 5.5 or later, you can utilize interval matching in switch statements. The syntax is concise and intuitive, making it easier to handle multiple cases within specific value ranges.
Basic Interval Matching
let score = 85
switch score {
case 0..<60:
print("You failed the exam.")
case 60..<70:
print("You got a D.")
case 70..<80:
print("You got a C.")
case 80..<90:
print("You got a B.")
case 90...100:
print("You got an A.")
default:
print("Invalid score.")
}
Half-Open vs. Closed Intervals
The half-open interval (using ..<
) does not include the upper bound, while the closed interval (using ...
) includes both the lower and upper bounds.
let value = 5
switch value {
case 0..<5:
print("Value is less than 5.")
case 5...10:
print("Value is between 5 and 10.")
default:
print("Value is greater than 10.")
}
Using where Clauses with Interval Matching
You can further enhance interval matching by using where
clauses to add additional conditions.
let temperature = 28
switch temperature {
case 0..<10 where temperature % 2 == 0:
print("Cold and even temperature.")
case 10..<20 where temperature % 2 != 0:
print("Cool and odd temperature.")
case 20..<30 where temperature % 2 == 0:
print("Warm and even temperature.")
default:
print("Hot temperature.")
}
0 Comment