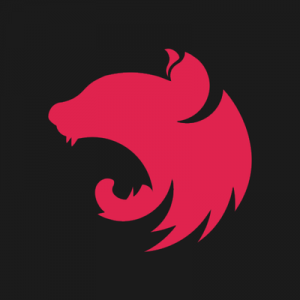
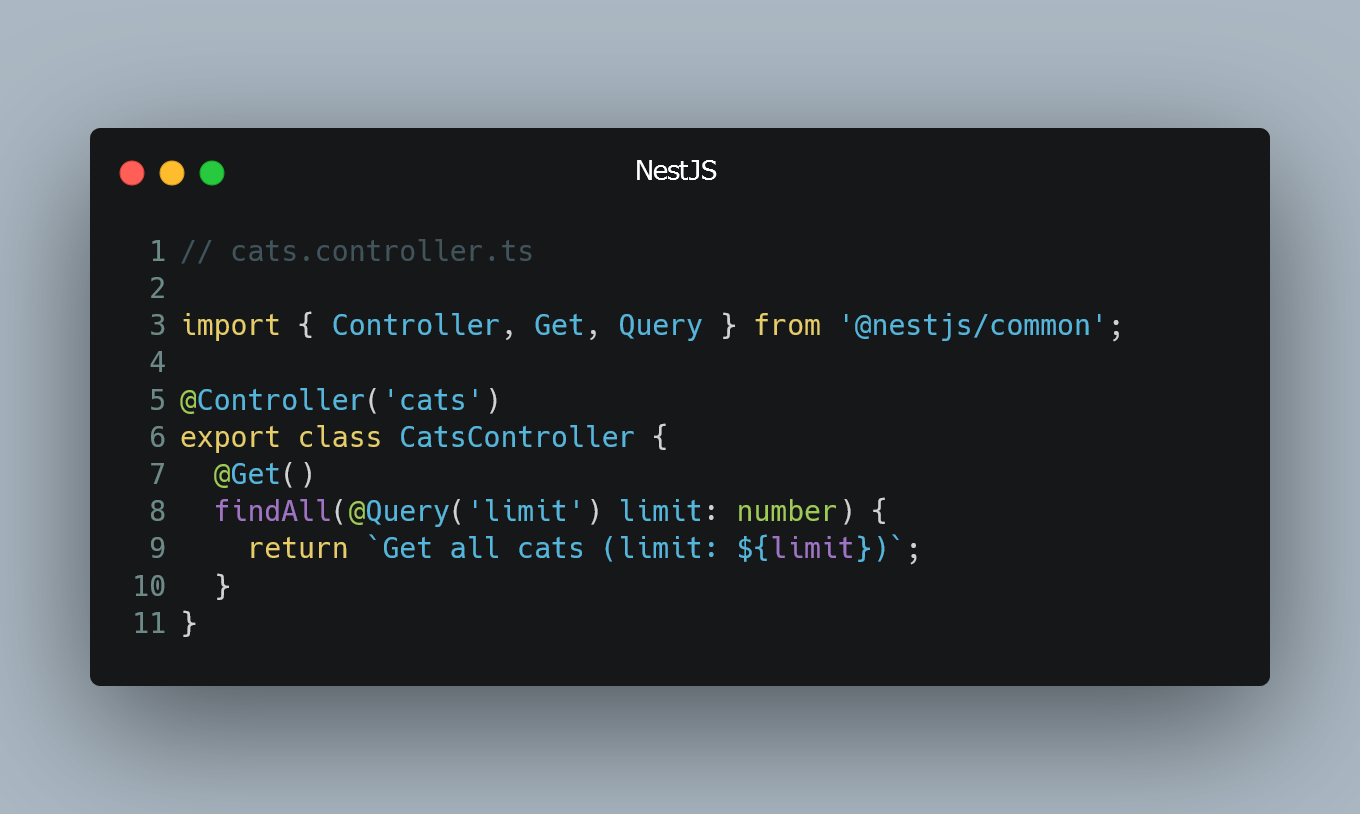
In Nest.js, query parameters are a common way to pass data to an API endpoint through the URL. They allow clients to send additional information to the server as part of the request.
Defining a Route with Query Parameters
To use query parameters, you need to define a route that can receive and handle them. In Nest.js, you can define routes using decorators such as @Get()
, @Post()
, @Put()
, etc. For this example, let's create a simple GET route with a query parameter.
// cats.controller.ts
import { Controller, Get, Query } from '@nestjs/common';
@Controller('cats')
export class CatsController {
@Get()
findAll(@Query('limit') limit: number) {
return `Get all cats (limit: ${limit})`;
}
}
In this example, we use the @Get()
decorator to define a GET route for the /cats
path. The findAll
method is the route handler that will be executed when a request is made to the /cats
endpoint. We use the @Query('limit')
decorator to extract the value of the limit
query parameter from the URL.
Sending a Request with Query Parameters
To send a request with query parameters, you can simply append them to the URL. For example, to send a request with the limit
query parameter set to 10
, you can make a GET request to /cats?limit=10
.
Handling Query Parameters in the Route Handler
In the route handler, you can access the query parameters using the variable names defined in the @Query()
decorators. In our example, we used the variable name limit
, so we can access the value of the limit
query parameter using the limit
variable.
The route handler can then use this value to perform any necessary logic, such as fetching data from a database with a limited number of results.
Handling Optional Query Parameters
Query parameters can be optional, meaning the client can choose whether to include them in the request or not. To handle optional query parameters, you can use the @Query()
decorator without specifying a variable name.
// cats.controller.ts
import { Controller, Get, Query } from '@nestjs/common';
@Controller('cats')
export class CatsController {
@Get()
findAll(@Query() query: any) {
const { limit, search } = query;
return `Get all cats (limit: ${limit}, search: ${search})`;
}
}
In this example, we don't specify a variable name in the @Query()
decorator. Instead, we receive all query parameters in the query
object. The route handler can then access the parameters using their names as properties of the query
object.
0 Comment