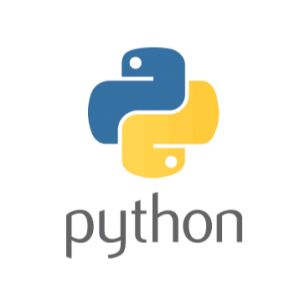
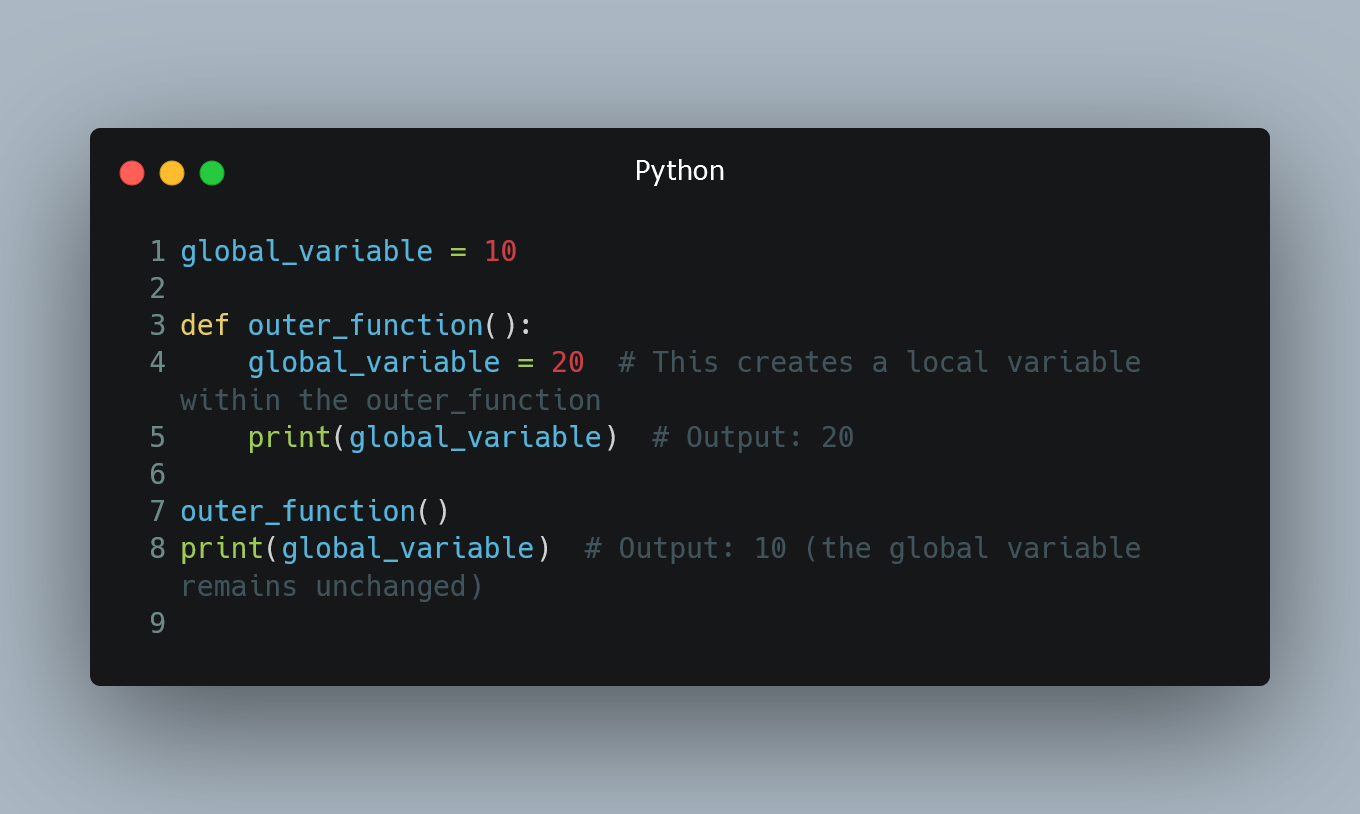
In Python, you can access and modify global variables from within a function. However, when modifying global variables inside a function, you need to be aware of the implications and use the global
keyword to indicate your intention explicitly.
#1. Accessing Global Variables
To access a global variable inside a function, simply refer to the variable by its name.
global_variable = 10
def access_global():
print(global_variable)
access_global() # Output: 10
In this example, the function access_global()
can access the value of the global variable global_variable
.
#2. Modifying Global Variables
If you want to modify the value of a global variable from within a function, you need to use the global
keyword to declare that you intend to use the global variable and not create a local variable with the same name.
global_variable = 10
def modify_global():
global global_variable
global_variable = 20
print(global_variable) # Output: 10
modify_global()
print(global_variable) # Output: 20
By using the global
keyword, you explicitly indicate that the variable being modified is the global one, not a local variable within the function.
#3. Caution with Global Variables
While it is possible to use global variables in functions, it's generally considered better practice to avoid extensive use of global variables. Modifying global variables from multiple functions can lead to code that is difficult to maintain and debug.
#4. Alternatives to Global Variables
Instead of relying heavily on global variables, consider passing variables as arguments to functions and returning values from functions when needed. This helps keep functions more self-contained and makes the code easier to understand.
#5. Global Variables in Nested Functions
When working with nested functions, be cautious when using global variables. A nested function can access a global variable from its enclosing scope without declaring it as global
. However, if the nested function attempts to modify the global variable, it will create a local variable instead.
global_variable = 10
def outer_function():
global_variable = 20 # This creates a local variable within the outer_function
print(global_variable) # Output: 20
outer_function()
print(global_variable) # Output: 10 (the global variable remains unchanged)
With the global
keyword, you can effectively use global variables inside functions, but it's important to use them judiciously and consider alternative approaches when possible.
0 Comment