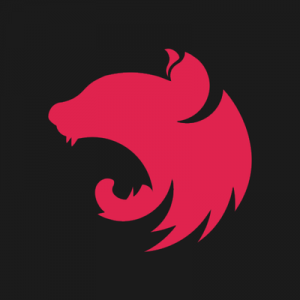
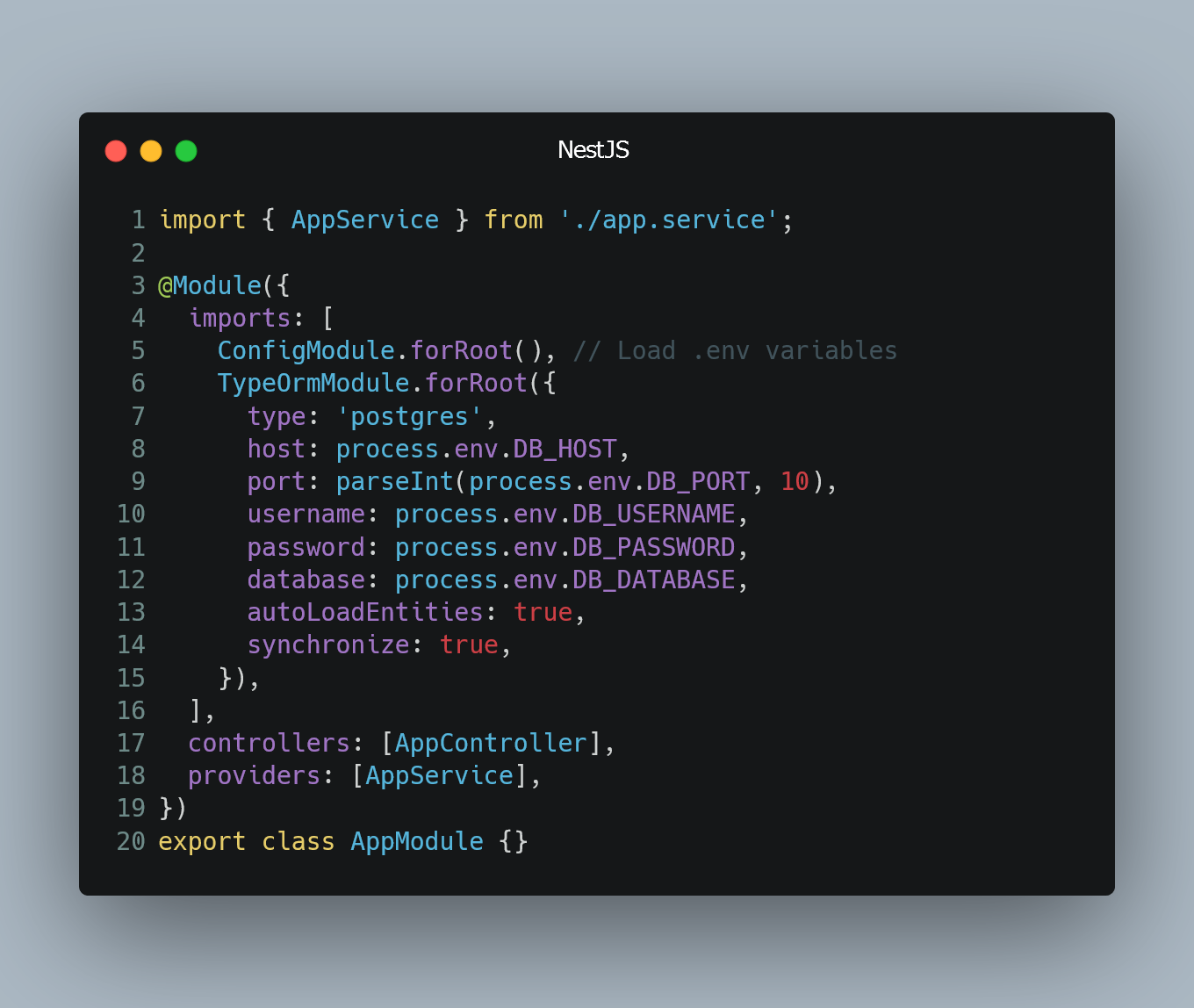
In NestJS, you can use .env variables to manage configuration settings, such as database connection details, in a more secure and flexible manner. This allows you to keep sensitive information separate from your codebase and easily switch between different configurations.
Setting Up dotenv Package
To use .env variables in your NestJS application, you need to install the dotenv
package, which allows you to read values from a .env file and make them accessible as environment variables.
First, install the dotenv
package as a development dependency in your NestJS project:
npm install dotenv --save-dev
Creating the .env File
Next, create a .env file in the root of your project directory. This file will contain the database connection settings as environment variables. For example:
DB_HOST=localhost
DB_PORT=5432
DB_USERNAME=myuser
DB_PASSWORD=mypassword
DB_DATABASE=mydatabase
Loading .env Variables in the Main App Module
To load the .env variables in the main app module file (e.g., app.module.ts
), you need to use the dotenv
package.
// app.module.ts
import { Module } from '@nestjs/common';
import { ConfigModule } from '@nestjs/config';
import { TypeOrmModule } from '@nestjs/typeorm';
import { AppController } from './app.controller';
import { AppService } from './app.service';
@Module({
imports: [
ConfigModule.forRoot(), // Load .env variables
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DB_HOST,
port: parseInt(process.env.DB_PORT, 10),
username: process.env.DB_USERNAME,
password: process.env.DB_PASSWORD,
database: process.env.DB_DATABASE,
autoLoadEntities: true,
synchronize: true,
}),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
In this example, we import the ConfigModule
from @nestjs/config
and use it in the imports
array of the main app module. This will load the .env variables and make them accessible through process.env
.
Accessing .env Variables
With the .env variables loaded, you can now access them in the main app module file or any other part of your NestJS application using process.env
. For example, in the TypeORM configuration above, we used process.env.DB_HOST
to get the database host value from the .env file.
0 Comment