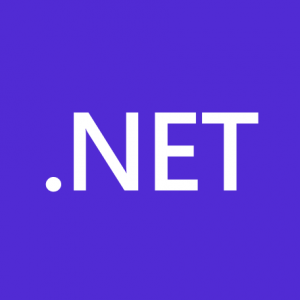
In C#, a NullReferenceException is a common runtime exception that occurs when you attempt to access or call a member (property, method, or field) of a null object reference.
What Causes a NullReferenceException?
A NullReferenceException is thrown when you try to perform an operation on an object reference that is currently set to null. Consider the following example:
string myString = null;
int length = myString.Length; // NullReferenceException will be thrown here
In this example, myString
is set to null, and when you try to access its Length
property, a NullReferenceException will be thrown because you cannot access a property of a null object.
Diagnosing a NullReferenceException
When a NullReferenceException occurs, the debugger will provide you with information about the line of code that triggered the exception. Look for the line number and identify which object reference is null.
Fixing a NullReferenceException
To fix a NullReferenceException, you need to ensure that the object reference you are working with is not null before accessing its members.
Option 1: Check for Null
You can use a simple null check using an if
statement before accessing the object's members:
if (myString != null)
{
int length = myString.Length; // No exception, only execute when myString is not null
}
Option 2: Null Conditional Operator (C# 6.0 and later)
You can use the null conditional operator (?.
) introduced in C# 6.0. It short-circuits the evaluation if the object reference is null:
int? length = myString?.Length; // length will be null if myString is null
Option 3: Initialize the Object
Make sure the object reference is properly initialized before accessing its members:
string myString = "Hello, World!";
int length = myString.Length; // No exception, myString is initialized
Null Coalescing Operator
Another useful operator is the null coalescing operator (??
). It allows you to provide a default value if the object reference is null:
string myString = null;
string result = myString ?? "Default Value"; // result will be "Default Value"
The null coalescing operator provides a fallback value when the object reference is null.
0 Comment