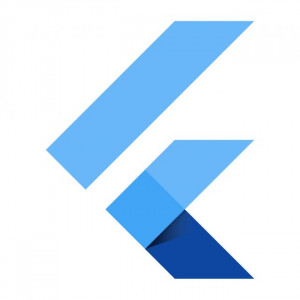
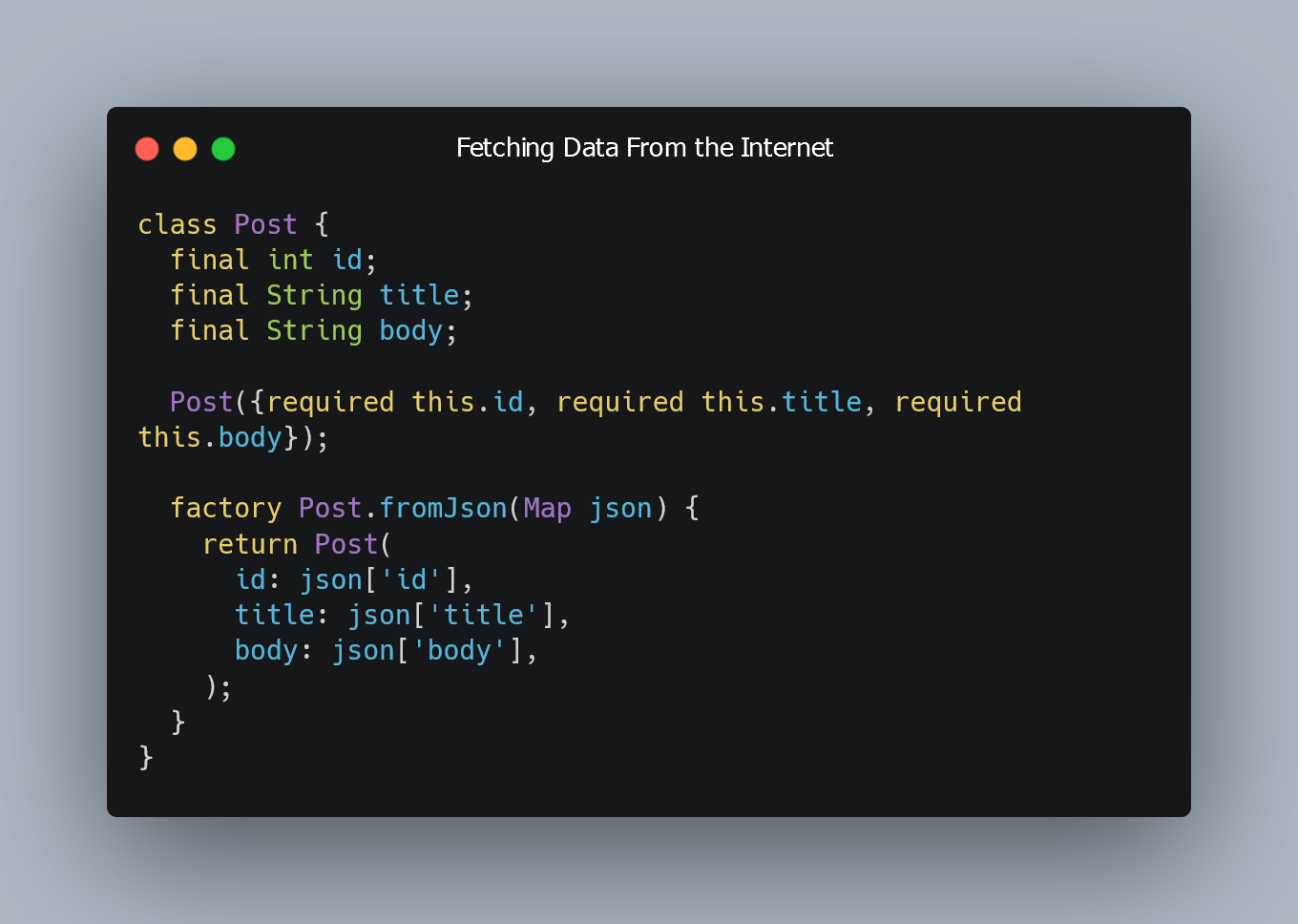
In Flutter, you can easily retrieve data from web APIs and display it in your app.
Setting Up the Project
Before we begin, ensure you have Flutter installed and set up on your system. Create a new Flutter project using the following command:
flutter create fetch_data_demo
Adding Dependencies
In your pubspec.yaml
file, add the http
package to your dependencies:
dependencies:
flutter:
sdk: flutter
http: ^0.14.0
Import the package:
import 'package:http/http.dart' as http;
Fetching Data From the Internet
Step 1: Create a Model Class
Create a model class to represent the data you'll be fetching. This class will help organize the data received from the API.
class Post {
final int id;
final String title;
final String body;
Post({required this.id, required this.title, required this.body});
factory Post.fromJson(Map json) {
return Post(
id: json['id'],
title: json['title'],
body: json['body'],
);
}
}
Step 2: Fetch Data From the API
Create a function to fetch data from the internet. In this example, we'll fetch data from the JSONPlaceholder API.
Future> fetchPosts() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
final List jsonResponse = json.decode(response.body);
return jsonResponse.map((data) => Post.fromJson(data)).toList();
} else {
throw Exception('Failed to load posts');
}
}
Step 3: Display the Data
In your widget's build
method, call the fetchPosts
function and display the fetched data.
class FetchDataWidget extends StatefulWidget {
@override
_FetchDataWidgetState createState() => _FetchDataWidgetState();
}
class _FetchDataWidgetState extends State {
late Future> futurePosts;
@override
void initState() {
super.initState();
futurePosts = fetchPosts();
}
@override
Widget build(BuildContext context) {
return FutureBuilder>(
future: futurePosts,
builder: (context, snapshot) {
if (snapshot.hasData) {
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(snapshot.data![index].title),
subtitle: Text(snapshot.data![index].body),
);
},
);
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return CircularProgressIndicator();
}
},
);
}
}
0 Comment