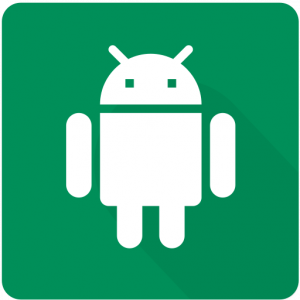
Published in
Android
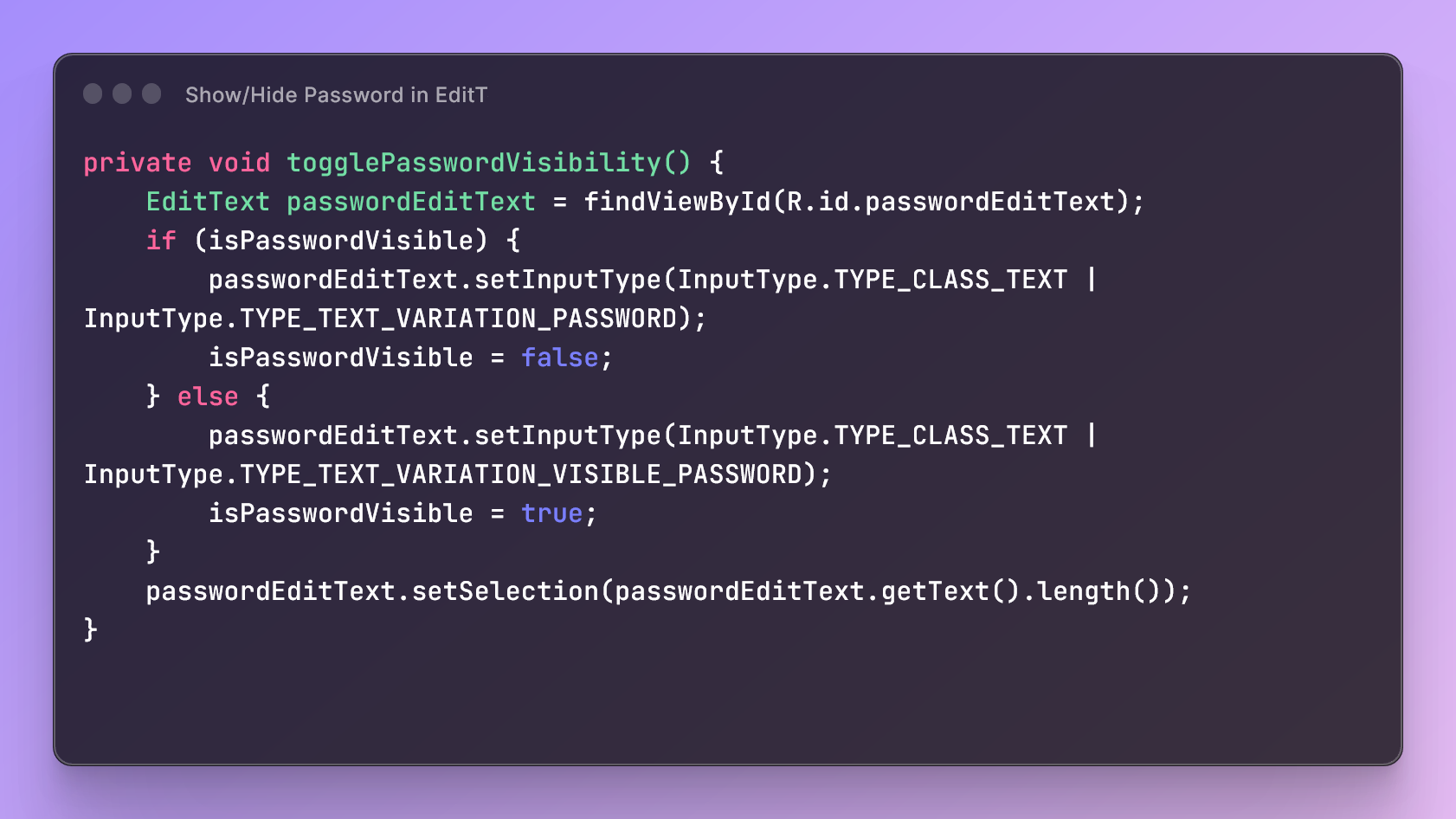
This feature allows users to toggle between displaying the password in plain text and hiding it behind asterisks or dots.
- Create an EditText in your layout XML file:
EditText
android:id="@+id/passwordEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="Enter your password"
- Define a boolean flag to track the password visibility state:
boolean isPasswordVisible = false;
- Create a method to toggle password visibility:
private void togglePasswordVisibility() {
EditText passwordEditText = findViewById(R.id.passwordEditText);
if (isPasswordVisible) {
// If password is visible, hide it by setting the input type to textPassword
passwordEditText.setInputType(InputType.TYPE_CLASS_TEXT | InputType.TYPE_TEXT_VARIATION_PASSWORD);
isPasswordVisible = false;
} else {
// If password is hidden, show it by setting the input type to text
passwordEditText.setInputType(InputType.TYPE_CLASS_TEXT | InputType.TYPE_TEXT_VARIATION_VISIBLE_PASSWORD);
isPasswordVisible = true;
}
// Move the cursor to the end of the text to maintain the current position
passwordEditText.setSelection(passwordEditText.getText().length());
}
- Set an OnClickListener to the EditText to toggle password visibility when a user clicks an eye icon or any other view:
EditText passwordEditText = findViewById(R.id.passwordEditText);
ImageView togglePasswordVisibilityImageView = findViewById(R.id.togglePasswordVisibilityImageView); // Replace with your eye icon or view
togglePasswordVisibilityImageView.setOnClickListener(v -> togglePasswordVisibility());
- You're done! Now, when the user clicks the eye icon or view, the password visibility will toggle between hidden and visible.
0 Comment