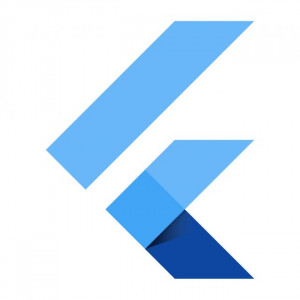
Published in
Flutter
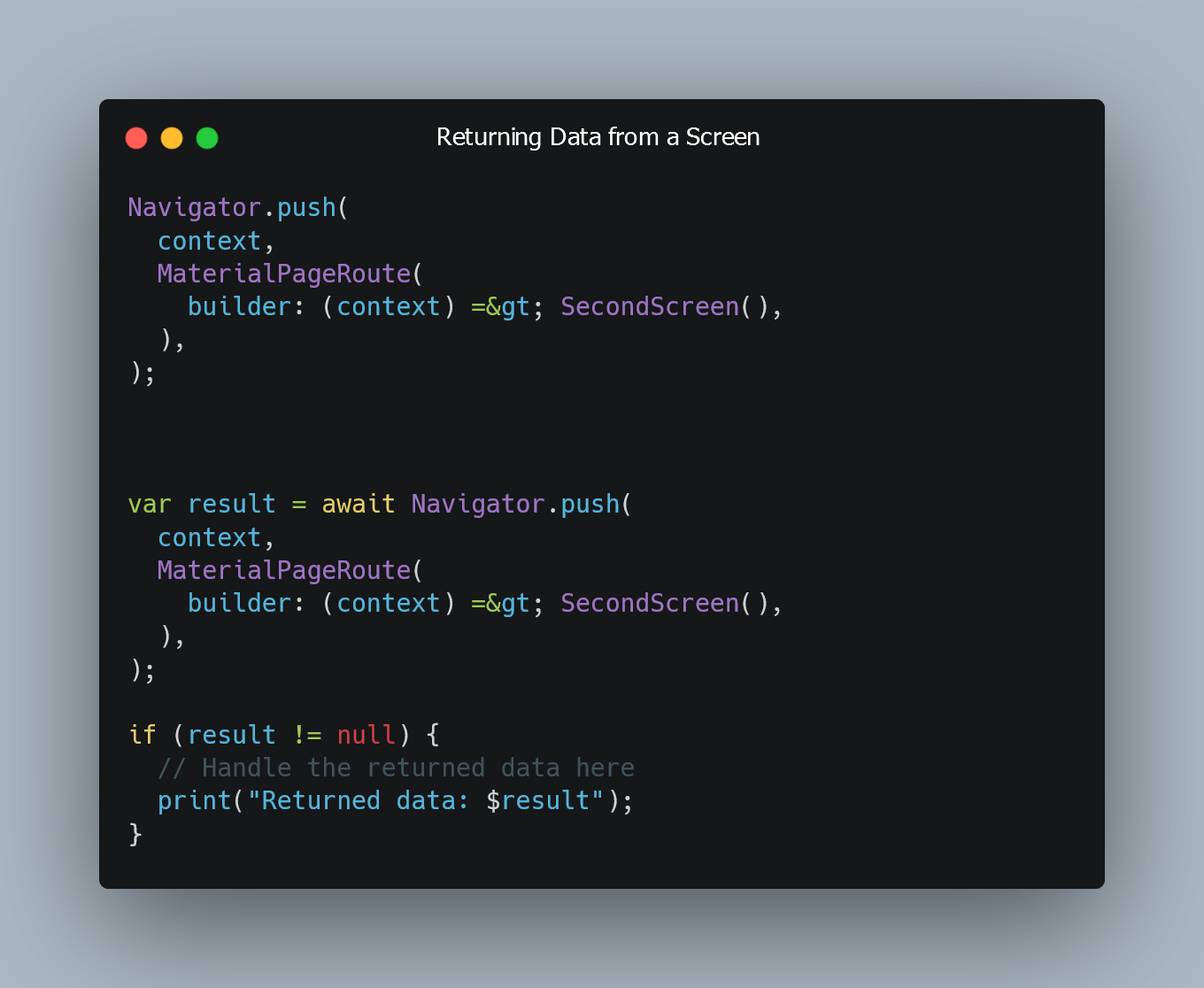
Explore how to return data from a screen in Flutter using Navigator.pop()
and await
.
Navigate to the Second Screen
In your first screen (the screen from which you want to receive data), use Navigator.push()
to navigate to the second screen.
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(),
),
);
Send Data Back from the Second Screen
In the second screen, when you want to return data to the first screen, use Navigator.pop()
with the data you want to pass back.
Navigator.pop(context, "Data to be returned");
Handle the Returned Data in the First Screen
In the first screen, use await
with Navigator.push()
to get the data returned from the second screen.
var result = await Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(),
),
);
if (result != null) {
// Handle the returned data here
print("Returned data: $result");
}
Step 4: Complete the Second Screen Navigation
Make sure to use Navigator.pop()
to complete the navigation in the second screen. This will return control to the first screen.
// Use Navigator.pop() to complete the navigation
Navigator.pop(context, "Data to be returned");
0 Comment