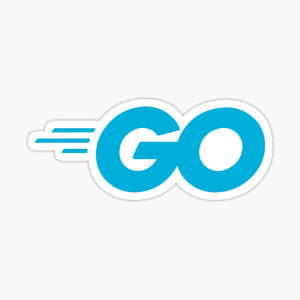
Published in
GO
In Go, there is no built-in enum type like in some other programming languages. However, there are idiomatic ways to represent enums using constants and custom types.
Using Constants
One way to represent enums in Go is by using a set of constants. By assigning unique values to each constant, you can create a set of distinct enum values.
Example
package main
import "fmt"
const (
Red = iota // 0
Green = iota // 1
Blue = iota // 2
)
func main() {
color := Green
switch color {
case Red:
fmt.Println("Color is Red")
case Green:
fmt.Println("Color is Green")
case Blue:
fmt.Println("Color is Blue")
default:
fmt.Println("Unknown Color")
}
}
Using iota with Custom Types
Another idiomatic approach to represent enums in Go is by using iota
with custom types. This allows you to create a more expressive and type-safe enum representation.
Example
package main
import "fmt"
type Color int
const (
Red Color = iota // 0
Green // 1
Blue // 2
)
func main() {
color := Green
switch color {
case Red:
fmt.Println("Color is Red")
case Green:
fmt.Println("Color is Green")
case Blue:
fmt.Println("Color is Blue")
default:
fmt.Println("Unknown Color")
}
}
0 Comment