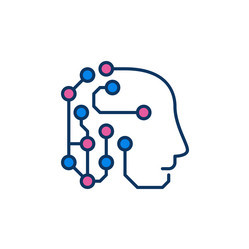
"Big O" notation is a way of describing the efficiency of an algorithm or the performance of a piece of code. It helps us understand how the runtime or space requirements of an algorithm grow as the input size increases. Here's a plain explanation of "Big O" notation:
Understanding Efficiency
When we write code or algorithms, we want them to be as efficient as possible. Efficiency refers to how quickly the code executes or how much memory it uses. As the size of the data (input) increases, we want our code to continue performing well without becoming too slow or using excessive memory.
Measuring Complexity
"Big O" notation provides a standardized way to measure the complexity of an algorithm or code. It allows us to compare different algorithms and see which one is more efficient for a given task.
Expressing Complexity
"Big O" notation expresses the complexity of an algorithm in terms of how its runtime or memory usage grows relative to the size of the input. It uses a mathematical notation, typically represented as O(f(n)), where 'f(n)' represents the function that describes the growth.
Common Notations
Some common "Big O" notations include:
- O(1) - Constant Time: The algorithm's performance does not change with the input size.
- O(log n) - Logarithmic Time: The performance grows slowly as the input size increases.
- O(n) - Linear Time: The performance scales linearly with the input size.
- O(n log n) - Log-Linear Time: The performance grows slightly faster than linearly.
- O(n^2) - Quadratic Time: The performance grows quadratically with the input size.
- O(2^n) - Exponential Time: The performance grows exponentially with the input size.
Focusing on Dominant Terms
In "Big O" notation, we focus on the dominant term, the term that grows the fastest, and ignore constant factors or lower-order terms. For example, if an algorithm has O(n^2 + n), we only consider the O(n^2) term.
The "Big O" Bound
"Big O" provides an upper bound on the growth of an algorithm. It tells us how an algorithm will behave at its worst but not necessarily its best. It helps us reason about scalability and understand the performance limits of our code.
0 Comment