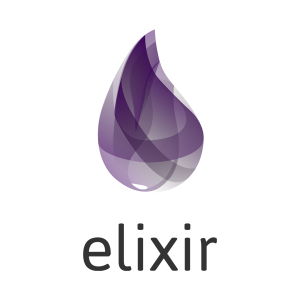
In Elixir, you can concatenate or join strings using various methods. Concatenating strings is a common operation in programming and can be achieved using different techniques.
Using String interpolation
String interpolation is a straightforward and commonly used method to join strings in Elixir. It allows you to embed variables or expressions directly within a string using the #{}
syntax.
Example:
first_name = "John"
last_name = "Doe"
full_name = "#{first_name} #{last_name}"
IO.puts(full_name) # Output: John Doe
In this example, we use string interpolation to concatenate the first_name
and last_name
variables into the full_name
string.
Using String Concatenation Operator
Elixir also provides the string concatenation operator <>
, which allows you to join strings explicitly.
Example:
greeting = "Hello, "
name = "Alice"
message = greeting <> name
IO.puts(message) # Output: Hello, Alice
In this example, we use the <>
operator to concatenate the greeting
and name
strings into the message
string.
Using Enum.join/2
If you have a list of strings that you want to join, you can use the Enum.join/2
function to concatenate them with a specified separator.
Example:
words = ["Elixir", "is", "awesome"]
sentence = Enum.join(words, " ")
IO.puts(sentence) # Output: Elixir is awesome
In this example, we use Enum.join/2
to concatenate the elements of the words
list into the sentence
string, separated by a space.
Using IO.puts with Multiple Arguments
The IO.puts/2
function in Elixir can accept multiple arguments, which will be printed on the same line.
Example:
name = "Bob"
age = 30
IO.puts("Name:", name, "Age:", age) # Output: Name: Bob Age: 30
In this example, we pass multiple arguments to IO.puts/2
to print the name
and age
variables on the same line without explicitly joining them.
0 Comment