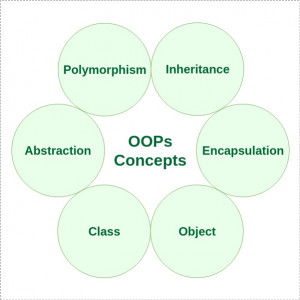
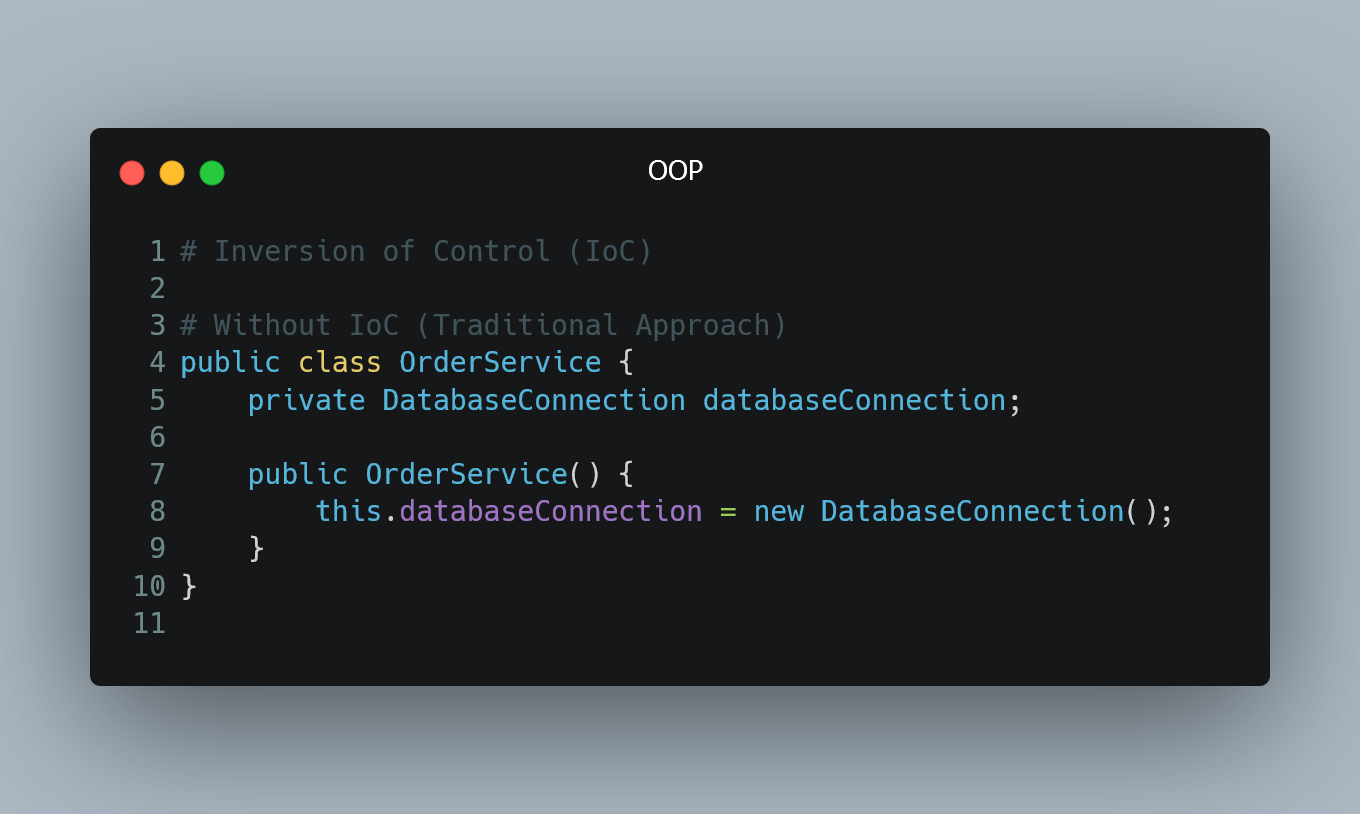
In software development, Inversion of Control (IoC) is a design principle that promotes decoupling and flexibility in code by shifting the control of the flow of execution from the main program to external components.
Understanding IoC
In traditional programming, the flow of control is determined by the main program. It creates objects, calls methods, and manages dependencies. This tight coupling can make the code harder to maintain, test, and extend.
With IoC, the control flow is inverted, giving control to external components or frameworks. These components are responsible for creating objects, resolving dependencies, and managing the flow of execution. The main program becomes more focused on the high-level logic and delegates the low-level details to external components.
Dependency Injection
A common way to implement IoC is through Dependency Injection (DI). Dependency Injection is a technique where an object's dependencies are provided by an external entity, rather than the object creating its dependencies itself.
Example:
// Without IoC (Traditional Approach)
public class OrderService {
private DatabaseConnection databaseConnection;
public OrderService() {
this.databaseConnection = new DatabaseConnection();
}
}
// With IoC (Dependency Injection)
public class OrderService {
private DatabaseConnection databaseConnection;
public OrderService(DatabaseConnection databaseConnection) {
this.databaseConnection = databaseConnection;
}
}
In the traditional approach, the OrderService
creates its own DatabaseConnection
. In the IoC approach using Dependency Injection, the DatabaseConnection
is provided by an external entity, such as a DI framework or a configuration file.
Benefits of IoC
-
Decoupling: IoC promotes loose coupling between components, making the code more modular and easier to maintain.
-
Testability: With external components managing dependencies, it becomes easier to mock and test individual components in isolation.
-
Flexibility: The code becomes more flexible and extensible, as changes to dependencies can be handled by the external components without modifying the main program.
0 Comment