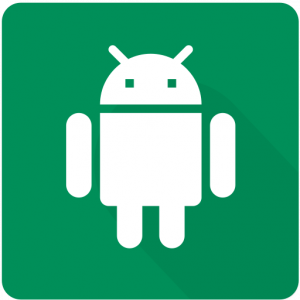
Published in
Android
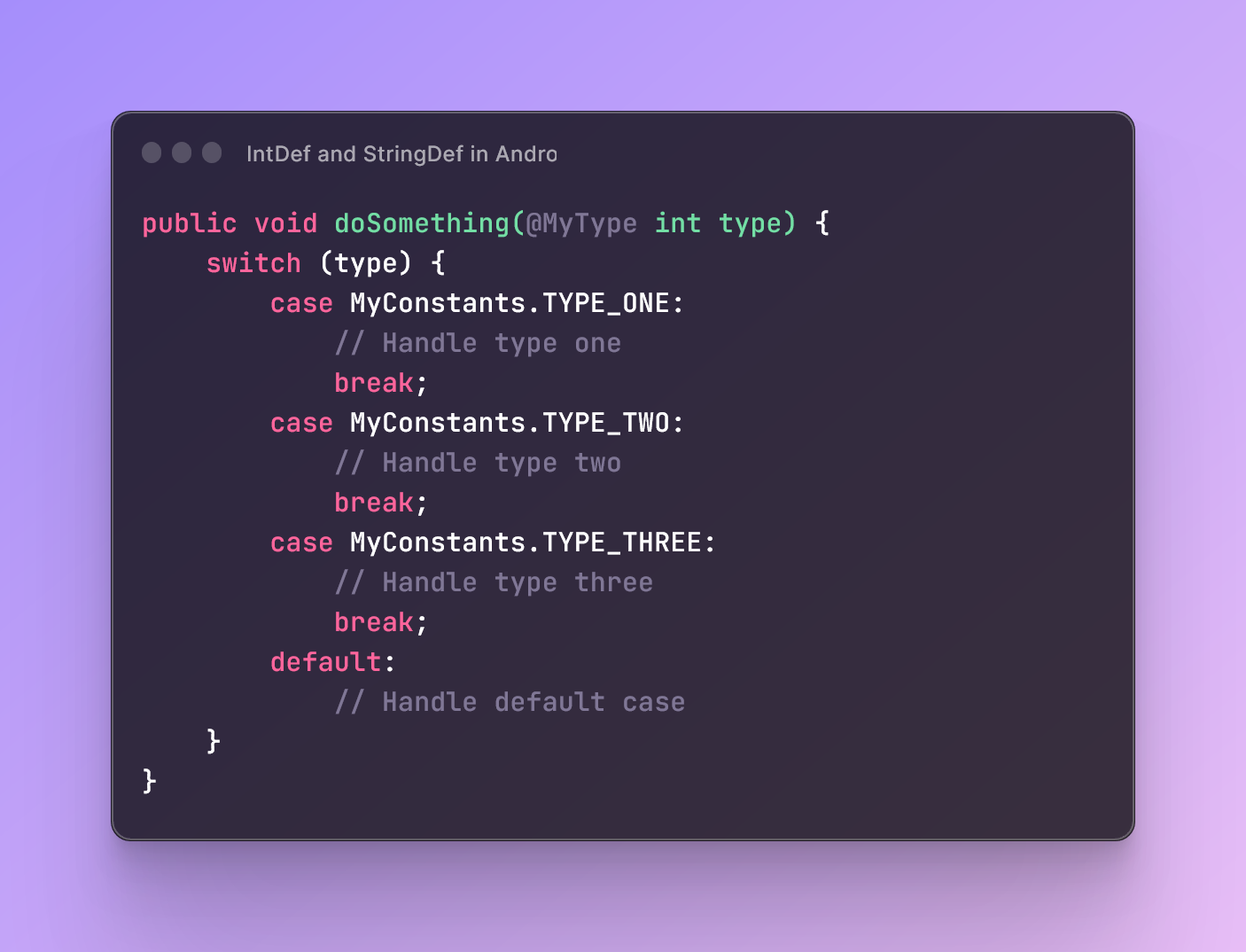
The IntDef
and StringDef
annotations in Android provide a powerful way to enforce compile-time type safety and improve code readability when using constants.
Using IntDef:
Define the Constants:
public class MyConstants {
public static final int TYPE_ONE = 1;
public static final int TYPE_TWO = 2;
public static final int TYPE_THREE = 3;
}
Annotate with IntDef:
import androidx.annotation.IntDef;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@IntDef({MyConstants.TYPE_ONE, MyConstants.TYPE_TWO, MyConstants.TYPE_THREE})
@Retention(RetentionPolicy.SOURCE)
public @interface MyType {}
Use the Constants:
public void doSomething(@MyType int type) {
switch (type) {
case MyConstants.TYPE_ONE:
// Handle type one
break;
case MyConstants.TYPE_TWO:
// Handle type two
break;
case MyConstants.TYPE_THREE:
// Handle type three
break;
default:
// Handle default case
}
}
Using StringDef:
Define the Constants:
public class MyConstants {
public static final String TYPE_ONE = "one";
public static final String TYPE_TWO = "two";
public static final String TYPE_THREE = "three";
}
Annotate with StringDef:
import androidx.annotation.StringDef;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@StringDef({MyConstants.TYPE_ONE, MyConstants.TYPE_TWO, MyConstants.TYPE_THREE})
@Retention(RetentionPolicy.SOURCE)
public @interface MyType {}
Use the Constants:
public void doSomething(@MyType String type) {
if (type.equals(MyConstants.TYPE_ONE)) {
// Handle type one
} else if (type.equals(MyConstants.TYPE_TWO)) {
// Handle type two
} else if (type.equals(MyConstants.TYPE_THREE)) {
// Handle type three
} else {
// Handle default case
}
}
Conclusion:
By using IntDef
and StringDef
, we can define and use constants in Android with increased compile-time safety and improved code readability. These annotations help prevent unintended use of incorrect values and make code maintenance more manageable. Leveraging IntDef
and StringDef
in your Android projects promotes better code organization and enhances overall code quality. Happy coding!
0 Comment