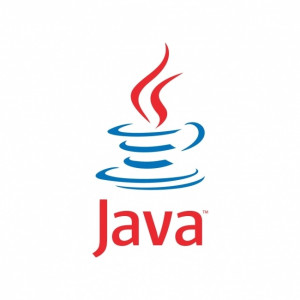
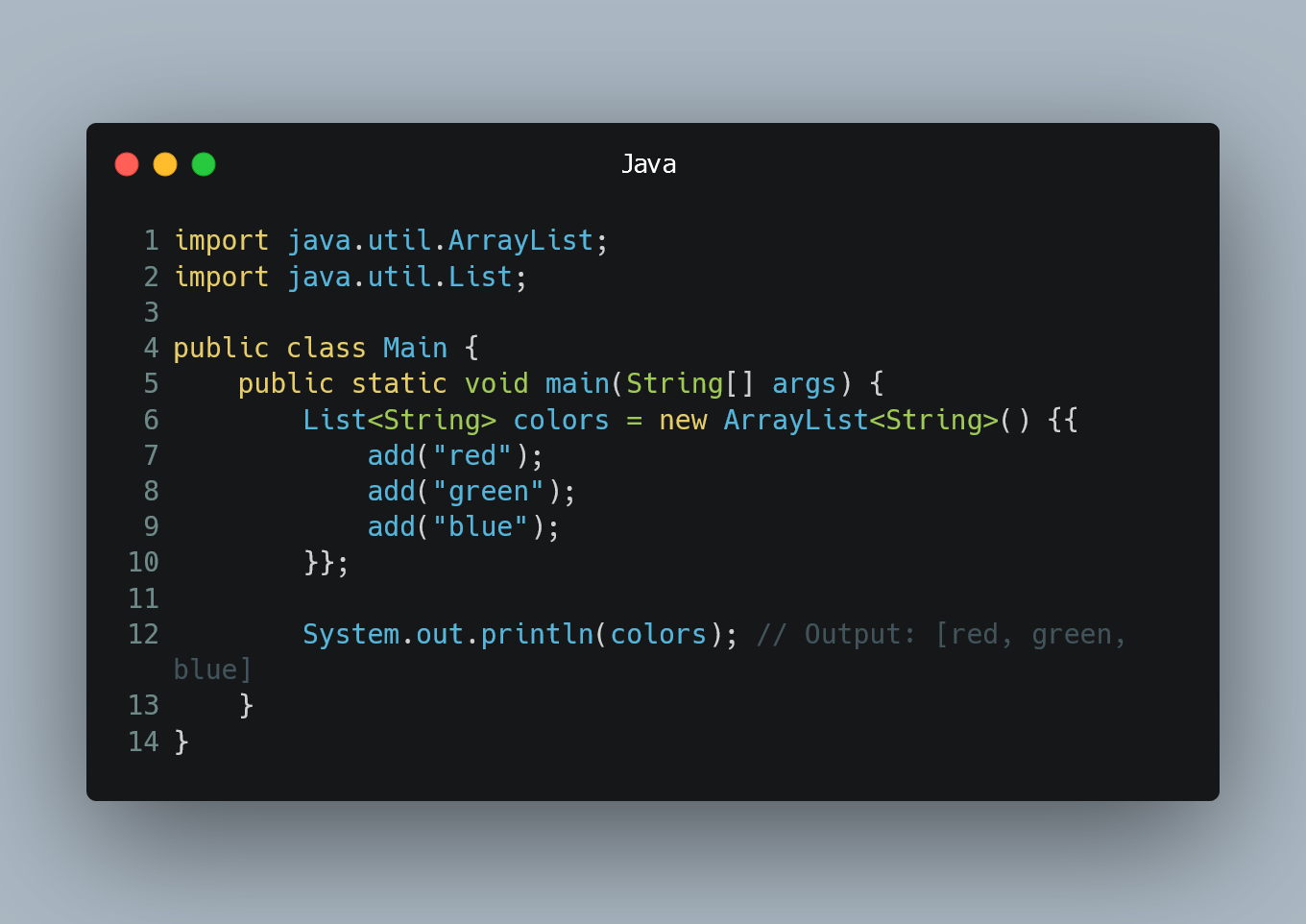
In Java, you can initialize an ArrayList in a concise one-liner using a feature known as "Double Brace Initialization" or by using the "Arrays.asList()" method.
Using Double Brace Initialization
Double Brace Initialization is a technique that combines an anonymous inner class with an instance initializer block to initialize a collection. It is a shorter alternative to traditional methods of initializing collections.
Example:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> colors = new ArrayList<String>() {{
add("red");
add("green");
add("blue");
}};
System.out.println(colors); // Output: [red, green, blue]
}
}
In this example, we use Double Brace Initialization to create and initialize an ArrayList of strings in a single line. The instance initializer block within the double braces allows us to add elements to the ArrayList without explicitly calling the add()
method.
Using Arrays.asList() (Java 9+)
Starting from Java 9, you can use the List.of()
method to create an immutable List in one line. However, if you need a mutable ArrayList, you can still use Arrays.asList()
and pass it to the ArrayList constructor.
Example:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> colors = new ArrayList<>(Arrays.asList("red", "green", "blue"));
System.out.println(colors); // Output: [red, green, blue]
}
}
In this example, we use Arrays.asList()
to create a List of strings containing "red", "green", and "blue", and then we pass it to the ArrayList constructor to initialize the ArrayList in one line.
0 Comment