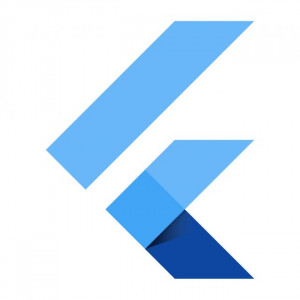
This layout is ideal for displaying a collection of items with different heights, such as images, cards, or tiles.
Setting Up the Project
Before we begin, ensure you have Flutter installed and set up on your system. Create a new Flutter project using the following command:
flutter create staggered_grid_view_demo
Adding Dependencies
In your pubspec.yaml
file, add the flutter_staggered_grid_view
package to your dependencies:
dependencies:
flutter:
sdk: flutter
flutter_staggered_grid_view: ^1.0.0
Import the package:
import 'package:flutter_staggered_grid_view/flutter_staggered_grid_view.dart';
Creating the Staggered Grid View
Step 1: Prepare the List of Items
Create a list of items that you want to display in the staggered grid view. For this example, we'll use a list of images.
List imageUrls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
// Add more image URLs as needed
];
Step 2: Build the Staggered Grid View Widget
In the build
method of your widget, create the staggered grid view using the StaggeredGridView.countBuilder
widget.
StaggeredGridView.countBuilder(
crossAxisCount: 2,
itemCount: imageUrls.length,
itemBuilder: (BuildContext context, int index) {
return Container(
decoration: BoxDecoration(
color: Colors.grey,
borderRadius: BorderRadius.circular(8.0),
),
child: Image.network(imageUrls[index], fit: BoxFit.cover),
);
},
staggeredTileBuilder: (int index) {
return StaggeredTile.count(1, index.isEven ? 1.2 : 1.5);
},
mainAxisSpacing: 8.0,
crossAxisSpacing: 8.0,
)
0 Comment