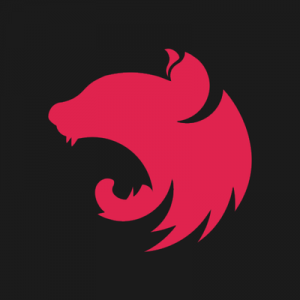
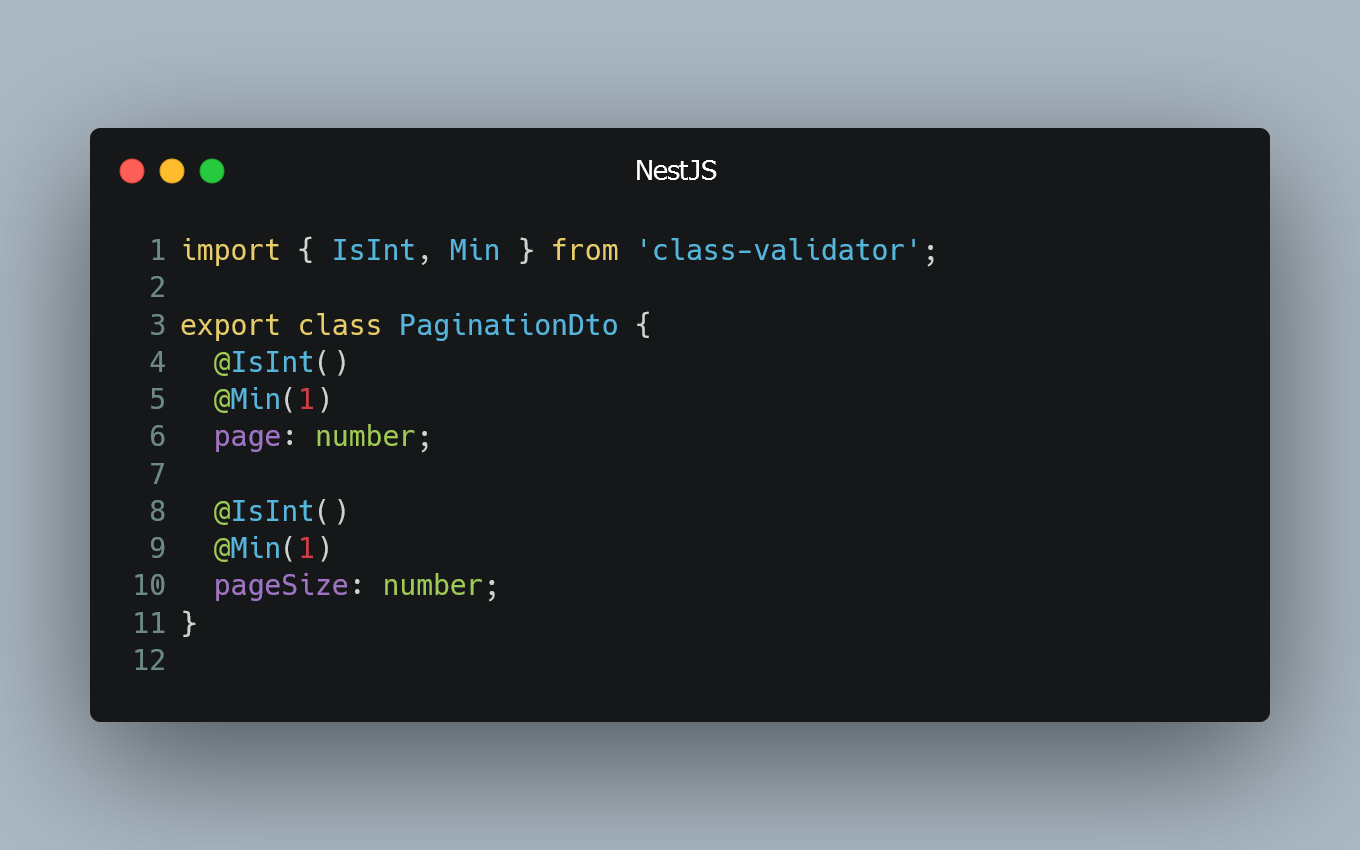
Pagination is a common requirement in APIs to manage large datasets. With NestJS and TypeORM, you can easily implement pagination to retrieve and display data in smaller, manageable chunks.
Setting Up the Database and TypeORM
First, make sure you have set up the database and TypeORM in your NestJS application. Ensure you have your entities defined and configured in the ormconfig.json
or ormconfig.js
file.
Defining Pagination Parameters
To implement pagination, you need to define pagination parameters such as the page number and the number of items per page. These parameters will be sent in the API request to specify which data to retrieve.
// pagination.dto.ts
import { IsInt, Min } from 'class-validator';
export class PaginationDto {
@IsInt()
@Min(1)
page: number;
@IsInt()
@Min(1)
pageSize: number;
}
In this example, we use a Data Transfer Object (DTO) named PaginationDto
to define the pagination parameters. The page
parameter represents the page number, and the pageSize
parameter represents the number of items per page.
Implementing Pagination in the Controller
Next, implement the pagination logic in your controller. You can use the findAndCount
method provided by TypeORM to retrieve paginated data.
// cats.controller.ts
import { Controller, Get, Query } from '@nestjs/common';
import { Cat } from './cat.entity';
import { PaginationDto } from './pagination.dto';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
@Controller('cats')
export class CatsController {
constructor(
@InjectRepository(Cat)
private readonly catRepository: Repository<Cat>,
) {}
@Get()
async findAll(@Query() paginationDto: PaginationDto): Promise<Cat[]> {
const { page, pageSize } = paginationDto;
const skip = (page - 1) * pageSize;
return this.catRepository.find({
take: pageSize,
skip,
});
}
}
In this example, we inject the Cat
repository from TypeORM using the @InjectRepository
decorator. In the findAll
method, we receive the PaginationDto
as a query parameter. We calculate the skip
value based on the page number and page size to skip the appropriate number of records in the query.
Sending Pagination Parameters
To use the pagination feature, send the page
and pageSize
parameters in the API request.
For example, to retrieve the second page with 10 items per page:
GET /cats?page=2&pageSize=10
The API will return the second page of cats with 10 cats per page.
0 Comment