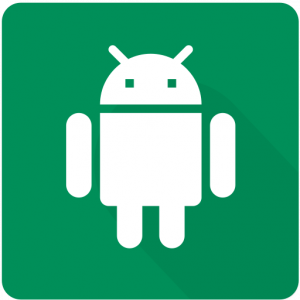
Published in
Android
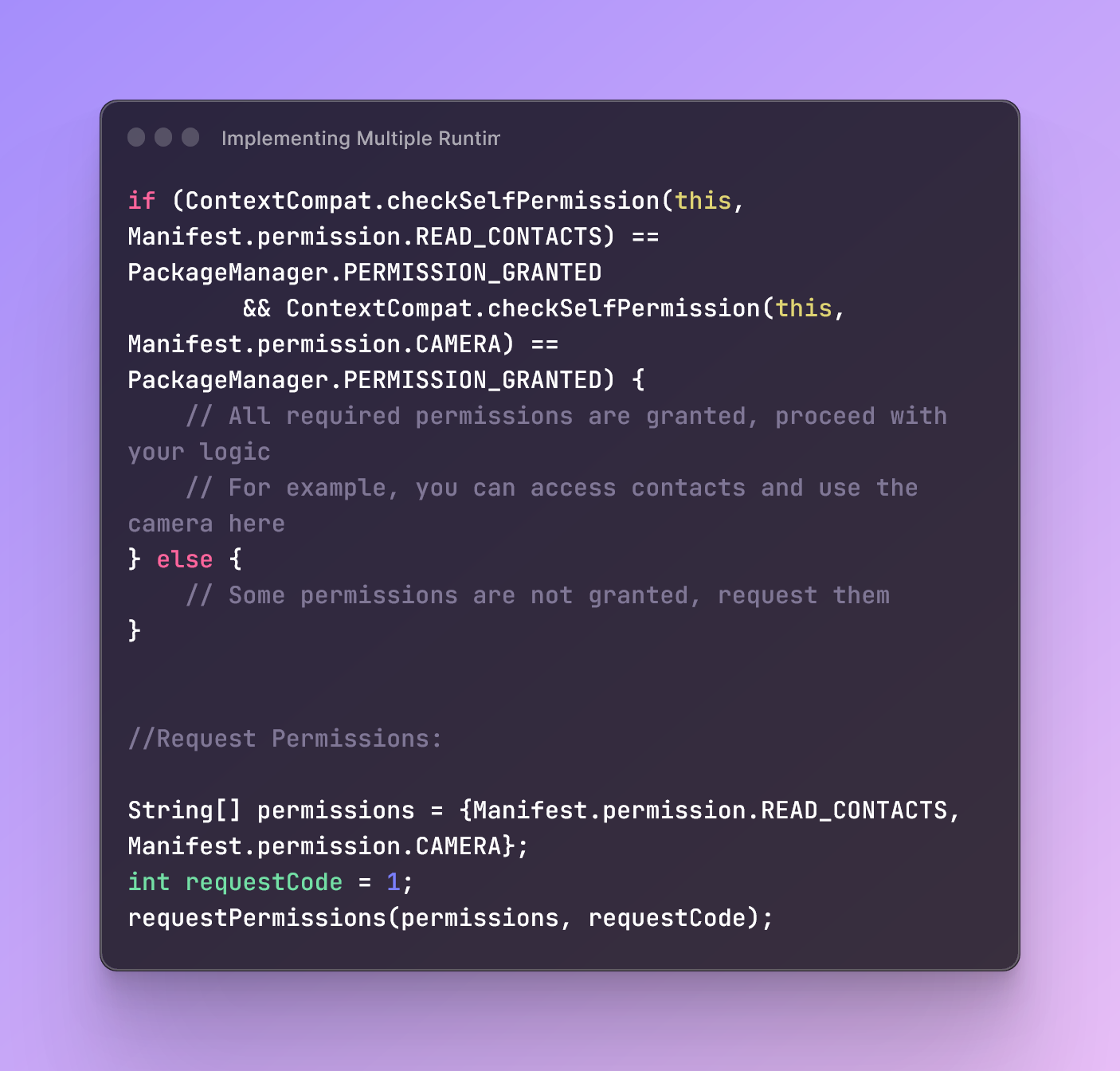
By following these steps, you can ensure your app complies with modern Android best practices for permission handling.
Check Permissions Availability:
Before requesting permissions, check if they are already granted using the checkSelfPermission
method:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.READ_CONTACTS) == PackageManager.PERMISSION_GRANTED
&& ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA) == PackageManager.PERMISSION_GRANTED) {
// All required permissions are granted, proceed with your logic
// For example, you can access contacts and use the camera here
} else {
// Some permissions are not granted, request them
}
Request Permissions:
When some permissions are not granted, request them using the requestPermissions
method:
String[] permissions = {Manifest.permission.READ_CONTACTS, Manifest.permission.CAMERA};
int requestCode = 1;
requestPermissions(permissions, requestCode);
Handle Permission Request Results:
Override the onRequestPermissionsResult
method to handle the permission request results:
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == 1) {
// Check if all permissions are granted
boolean allPermissionsGranted = true;
for (int result : grantResults) {
if (result != PackageManager.PERMISSION_GRANTED) {
allPermissionsGranted = false;
break;
}
}
if (allPermissionsGranted) {
// All permissions are granted, proceed with your logic
} else {
// Some permissions are denied, handle this situation (e.g., show a message or disable functionality)
}
}
}
Handle "Never Ask Again":
If a user selects "Never ask again" while denying a permission, you can guide them to the app settings screen using the shouldShowRequestPermissionRationale
method:
if (!shouldShowRequestPermissionRationale(Manifest.permission.READ_CONTACTS)
|| !shouldShowRequestPermissionRationale(Manifest.permission.CAMERA)) {
// User has selected "Never ask again," show a message explaining why the permission is required and direct them to app settings
}
0 Comment