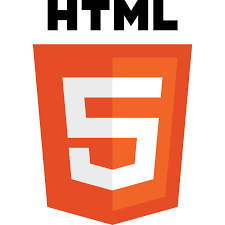
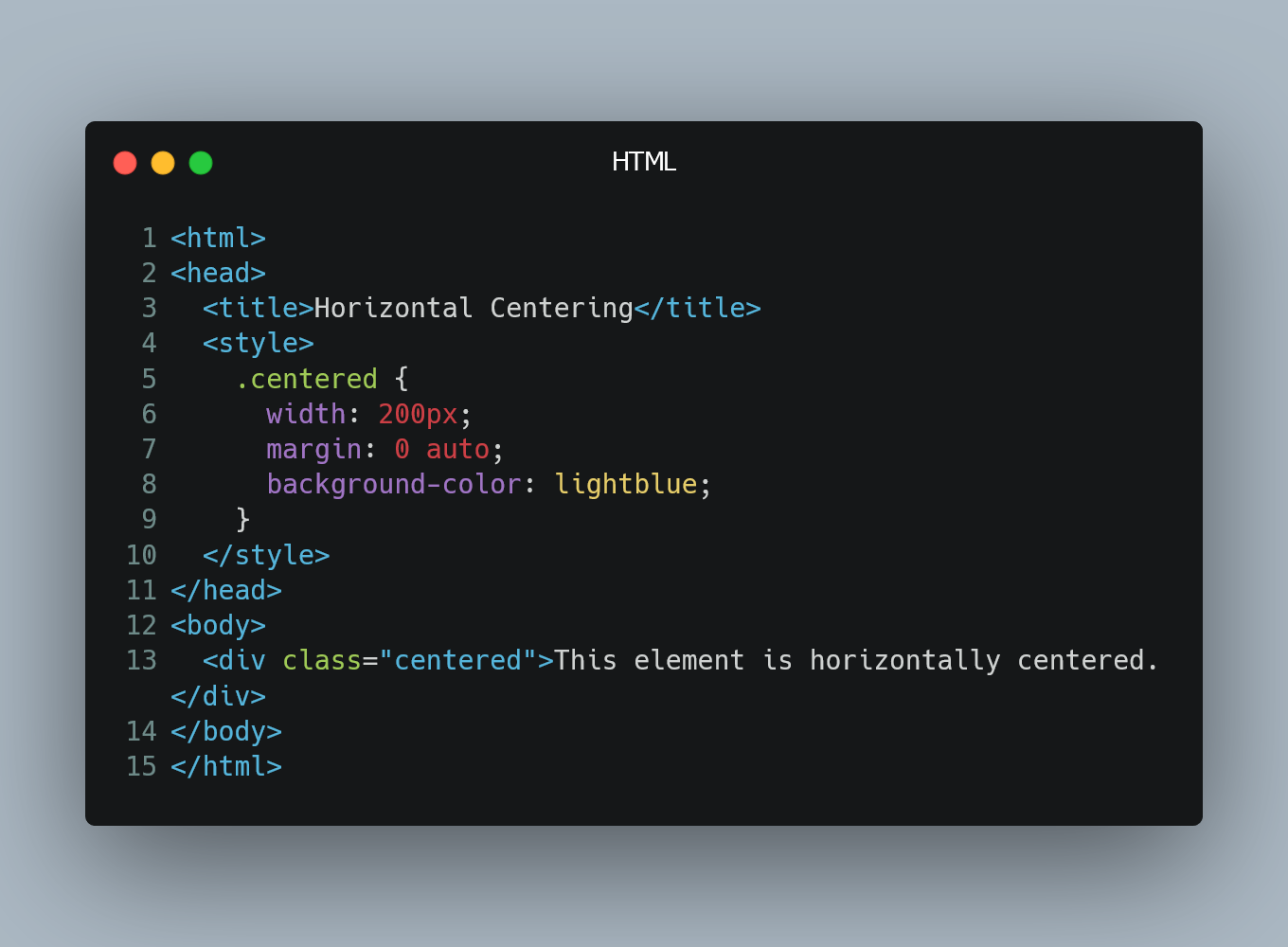
To horizontally center an element in HTML and CSS, you can use various techniques depending on the layout and positioning requirements. Here are some commonly used methods:
#1. Using Margin: Auto
The simplest way to horizontally center a block-level element is by using the margin: auto
CSS property.
<!DOCTYPE html>
<html>
<head>
<title>Horizontal Centering</title>
<style>
.centered {
width: 200px;
margin: 0 auto;
background-color: lightblue;
}
</style>
</head>
<body>
<div class="centered">This element is horizontally centered.</div>
</body>
</html>
In this example, the .centered
class has a specified width and uses margin: 0 auto
to center the element horizontally within its parent container.
#2. Using Flexbox
Flexbox is a powerful layout model that makes centering elements straightforward.
<!DOCTYPE html>
<html>
<head>
<title>Horizontal Centering</title>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 300px;
}
.centered {
background-color: lightblue;
}
</style>
</head>
<body>
<div class="container">
<div class="centered">This element is horizontally centered using Flexbox.</div>
</div>
</body>
</html>
In this example, the .container
class uses display: flex
to create a flex container. The justify-content: center
and align-items: center
properties are used to center the child element horizontally and vertically within the container.
#3. Using Grid
If you're working with CSS Grid, you can use grid properties to center elements.
<!DOCTYPE html>
<html>
<head>
<title>Horizontal Centering</title>
<style>
.container {
display: grid;
place-items: center;
height: 300px;
}
.centered {
background-color: lightblue;
}
</style>
</head>
<body>
<div class="container">
<div class="centered">This element is horizontally centered using CSS Grid.</div>
</div>
</body>
</html>
In this example, the .container
class uses display: grid
and place-items: center
to center the child element within the container.
#4. Using Positioning and Transforms
For more complex layouts or absolute positioning, you can use position: absolute
and transform
to center an element.
<!DOCTYPE html>
<html>
<head>
<title>Horizontal Centering</title>
<style>
.container {
position: relative;
height: 300px;
}
.centered {
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
background-color: lightblue;
}
</style>
</head>
<body>
<div class="container">
<div class="centered">This element is horizontally centered using positioning and transforms.</div>
</div>
</body>
</html>
In this example, the .centered
class uses absolute positioning and transform: translate(-50%, -50%)
to center the element within its relative-positioned parent container.
Choose the method that best suits your layout and styling needs when horizontally centering an element in HTML and CSS. Each approach offers different flexibility and can be adapted based on your specific use case.
0 Comment