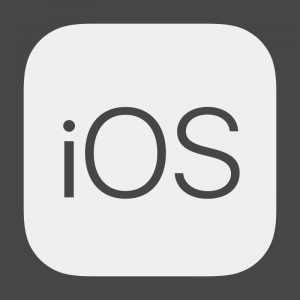
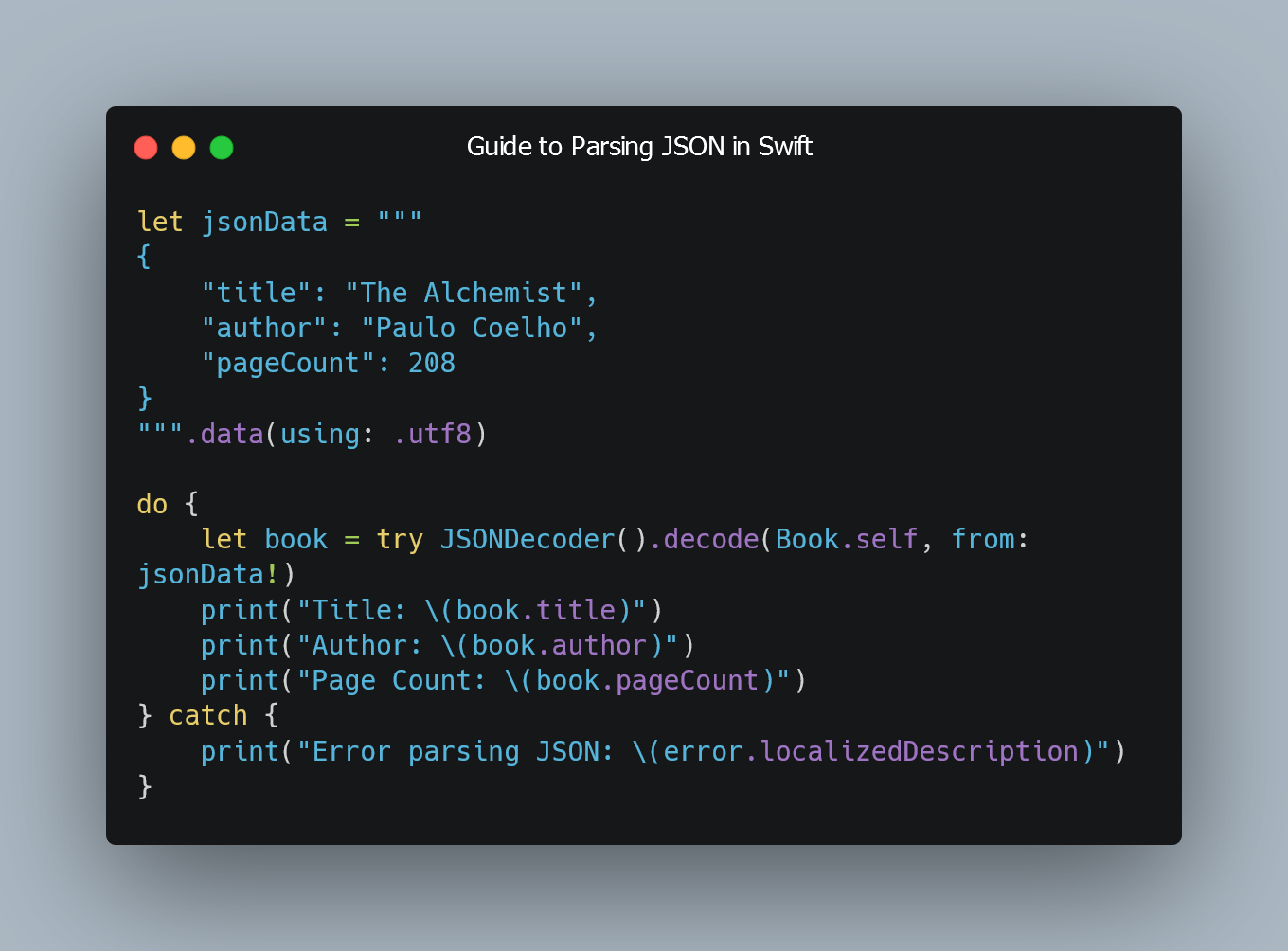
In Swift, parsing JSON is a common task when working with web APIs or data fetched from the server.
JSON Structure
Before parsing JSON, it's essential to understand its structure. JSON data consists of key-value pairs, arrays, and nested objects.
Decodable Protocol
Swift provides the Decodable
protocol to facilitate parsing JSON into Swift native data types. To parse JSON using Decodable
, create a corresponding Swift struct or class that conforms to the Decodable
protocol. Each property of the struct should match the JSON key, and the data type should match the JSON value.
Parsing JSON with Decodable
struct Book: Decodable {
let title: String
let author: String
let pageCount: Int
}
let jsonData = """
{
"title": "The Alchemist",
"author": "Paulo Coelho",
"pageCount": 208
}
""".data(using: .utf8)
do {
let book = try JSONDecoder().decode(Book.self, from: jsonData!)
print("Title: \(book.title)")
print("Author: \(book.author)")
print("Page Count: \(book.pageCount)")
} catch {
print("Error parsing JSON: \(error.localizedDescription)")
}
Nested Objects and Arrays
For JSON with nested objects or arrays, simply create corresponding structs or classes for each level of nesting, and Swift's Decodable
protocol will handle the rest.
Parsing JSON with Nested Objects
struct Address: Decodable {
let city: String
let country: String
}
struct Person: Decodable {
let name: String
let age: Int
let address: Address
}
let jsonData = """
{
"name": "John Doe",
"age": 30,
"address": {
"city": "New York",
"country": "USA"
}
}
""".data(using: .utf8)
do {
let person = try JSONDecoder().decode(Person.self, from: jsonData!)
print("Name: \(person.name)")
print("Age: \(person.age)")
print("Address: \(person.address.city), \(person.address.country)")
} catch {
print("Error parsing JSON: \(error.localizedDescription)")
}
Parsing JSON with Arrays
struct Product: Decodable {
let name: String
let price: Double
}
let jsonData = """
[
{
"name": "iPhone 12",
"price": 799
},
{
"name": "AirPods Pro",
"price": 249
}
]
""".data(using: .utf8)
do {
let products = try JSONDecoder().decode([Product].self, from: jsonData!)
for product in products {
print("Name: \(product.name), Price: \(product.price)")
}
} catch {
print("Error parsing JSON: \(error.localizedDescription)")
}
0 Comment