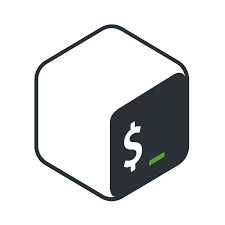
Published in
Bash
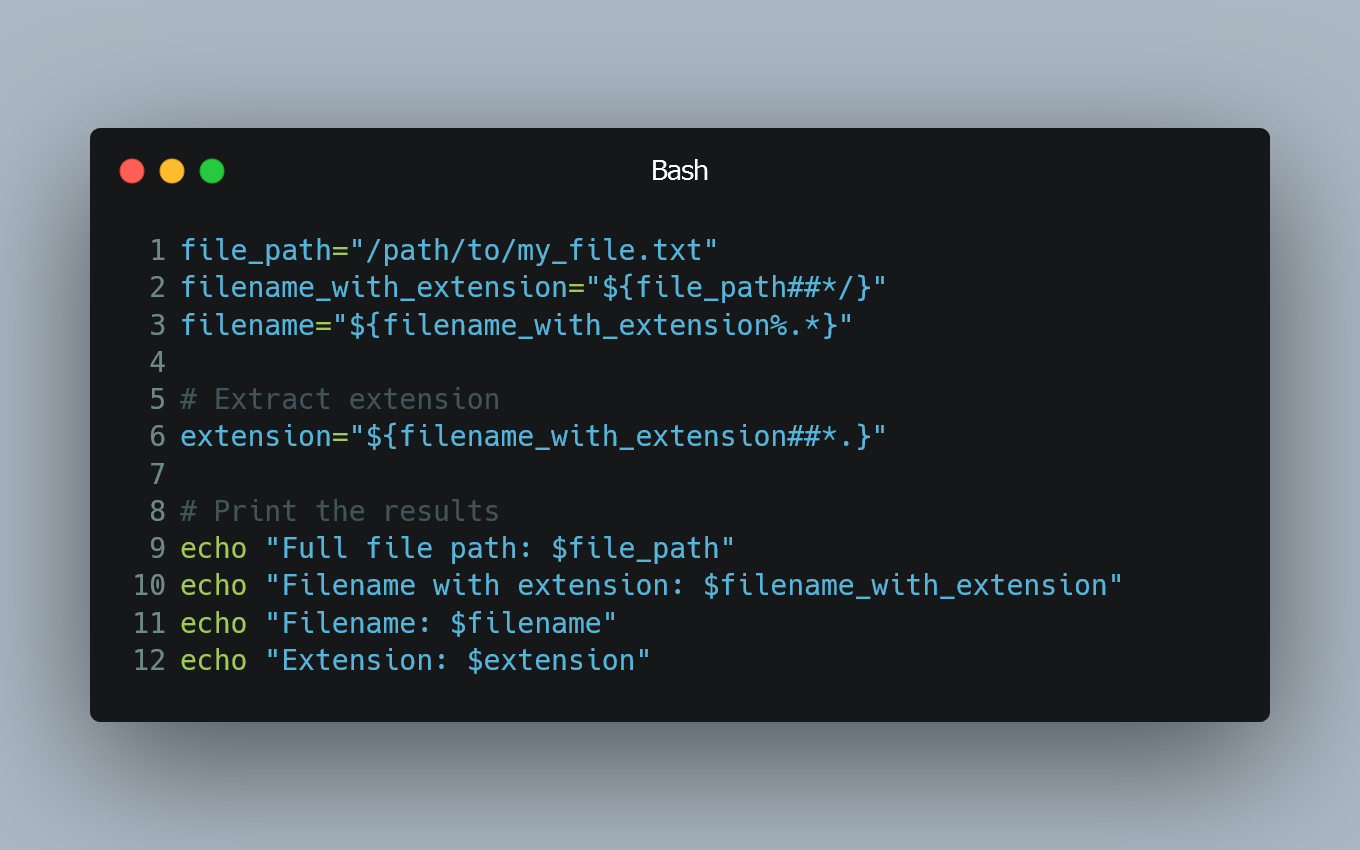
To extract the filename and extension from a file path in Bash, you can use various string manipulation techniques.
Using parameter expansion
Parameter expansion allows you to manipulate variables and extract the filename and extension.
# Example file path
file_path="/path/to/my_file.txt"
# Extract filename with extension
filename_with_extension="${file_path##*/}"
# Extract filename without extension
filename="${filename_with_extension%.*}"
# Extract extension
extension="${filename_with_extension##*.}"
# Print the results
echo "Full file path: $file_path"
echo "Filename with extension: $filename_with_extension"
echo "Filename: $filename"
echo "Extension: $extension"
Using basename
command
The basename
command can be used to extract the filename with or without the extension.
# Example file path
file_path="/path/to/my_file.txt"
# Extract filename with extension
filename_with_extension=$(basename "$file_path")
# Extract filename without extension
filename="${filename_with_extension%.*}"
# Extract extension
extension="${filename_with_extension##*.}"
# Print the results
echo "Full file path: $file_path"
echo "Filename with extension: $filename_with_extension"
echo "Filename: $filename"
echo "Extension: $extension"
Using dirname
command
The dirname
command can be used to extract the directory path.
# Example file path
file_path="/path/to/my_file.txt"
# Extract directory path
directory_path=$(dirname "$file_path")
# Extract filename with extension
filename_with_extension=$(basename "$file_path")
# Extract filename without extension
filename="${filename_with_extension%.*}"
# Extract extension
extension="${filename_with_extension##*.}"
# Print the results
echo "Full file path: $file_path"
echo "Directory path: $directory_path"
echo "Filename with extension: $filename_with_extension"
echo "Filename: $filename"
echo "Extension: $extension"
Using rev
command (GNU coreutils)
If you have access to the rev
command, you can use it to reverse the string and then extract the filename and extension.
# Example file path
file_path="/path/to/my_file.txt"
# Reverse the file path
reversed=$(echo "$file_path" | rev)
# Extract filename with extension (reversed)
filename_with_extension="${reversed%%/*}"
# Reverse the filename with extension back to normal
filename_with_extension=$(echo "$filename_with_extension" | rev)
# Extract filename without extension
filename="${filename_with_extension%.*}"
# Extract extension
extension="${filename_with_extension##*.}"
# Print the results
echo "Full file path: $file_path"
echo "Filename with extension: $filename_with_extension"
echo "Filename: $filename"
echo "Extension: $extension"
0 Comment