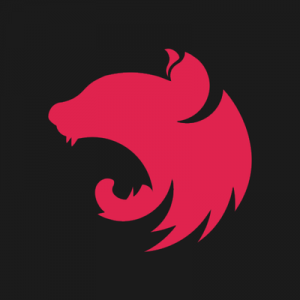
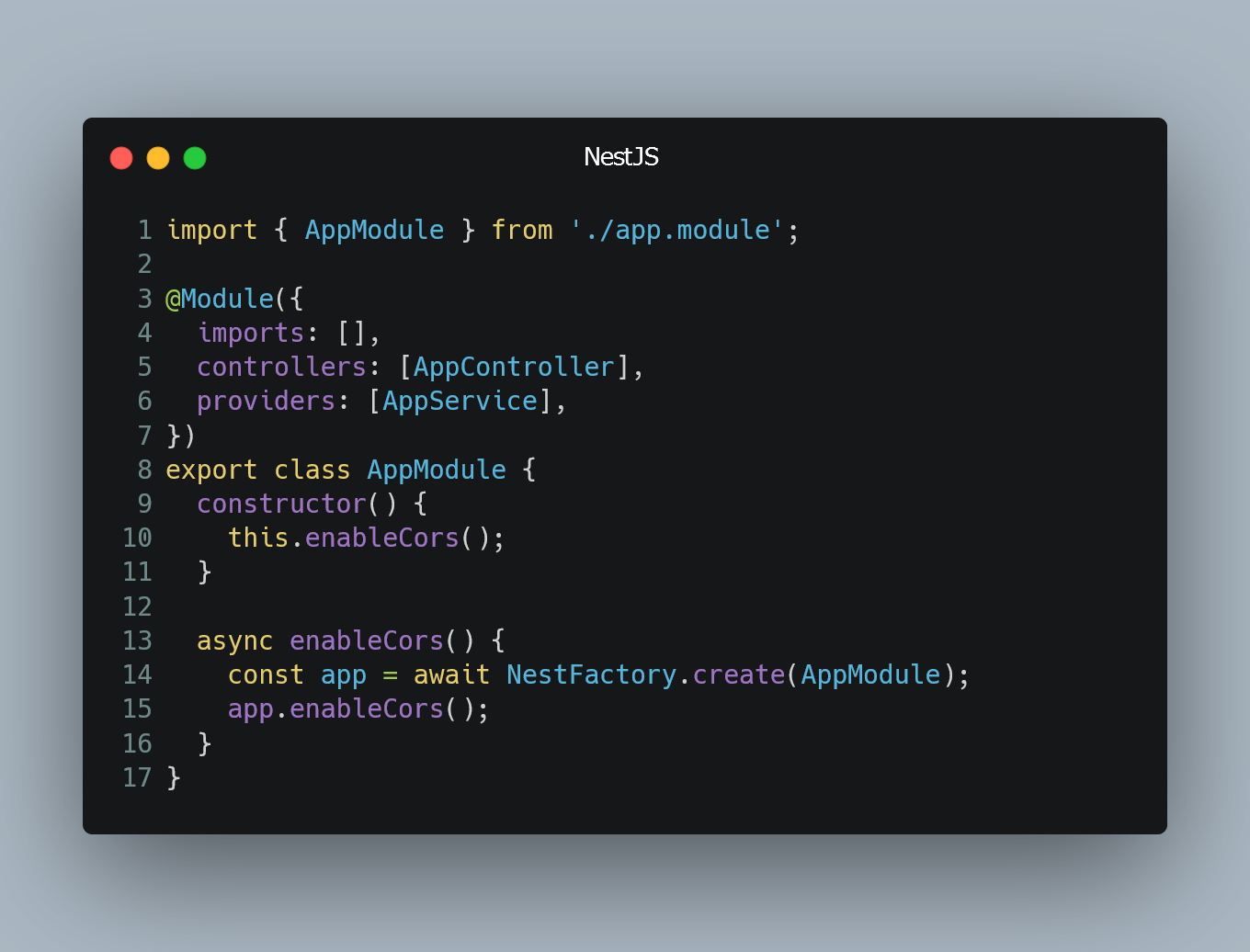
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers that restricts web pages from making requests to a different domain than the one that served the web page.
Install the @nestjs/platform-express package
First, ensure that you have the @nestjs/platform-express
package installed in your NestJS project.
npm install @nestjs/platform-express --save
Enabling CORS
In your main application module (usually app.module.ts
), you can enable CORS by using the enableCors()
method of the Nest application instance.
// app.module.ts
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
@Module({
imports: [],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {
constructor() {
this.enableCors();
}
async enableCors() {
const app = await NestFactory.create(AppModule);
app.enableCors();
}
}
In this example, we use the enableCors()
method inside the constructor of the AppModule
to enable CORS. The enableCors()
method adds the necessary headers to allow cross-origin requests.
Configuring CORS Options
Optionally, you can pass configuration options to the enableCors()
method to customize the CORS behavior. The available configuration options include:
-
origin
: A string or an array of strings representing allowed origins. -
methods
: A string or an array of strings representing allowed HTTP methods. -
allowedHeaders
: A string or an array of strings representing allowed headers. -
exposedHeaders
: A string or an array of strings representing headers exposed to the client. -
credentials
: A boolean value indicating whether to include credentials in the request (e.g., cookies, HTTP authentication). -
maxAge
: A number representing the maximum age of the preflight request (in seconds).
// app.module.ts
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
@Module({
imports: [],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {
constructor() {
this.enableCors();
}
async enableCors() {
const app = await NestFactory.create(AppModule);
app.enableCors({
origin: 'https://example.com',
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization'],
credentials: true,
maxAge: 86400,
});
}
}
In this example, we pass the enableCors()
method an options object with custom configuration settings. We allow requests from 'https://example.com', permit only GET and POST methods, specify allowed headers, include credentials, and set a maximum age of 24 hours for preflight requests.
0 Comment