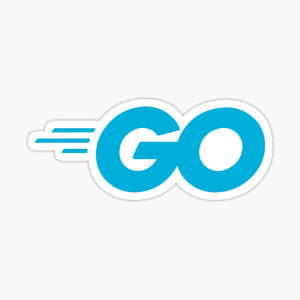
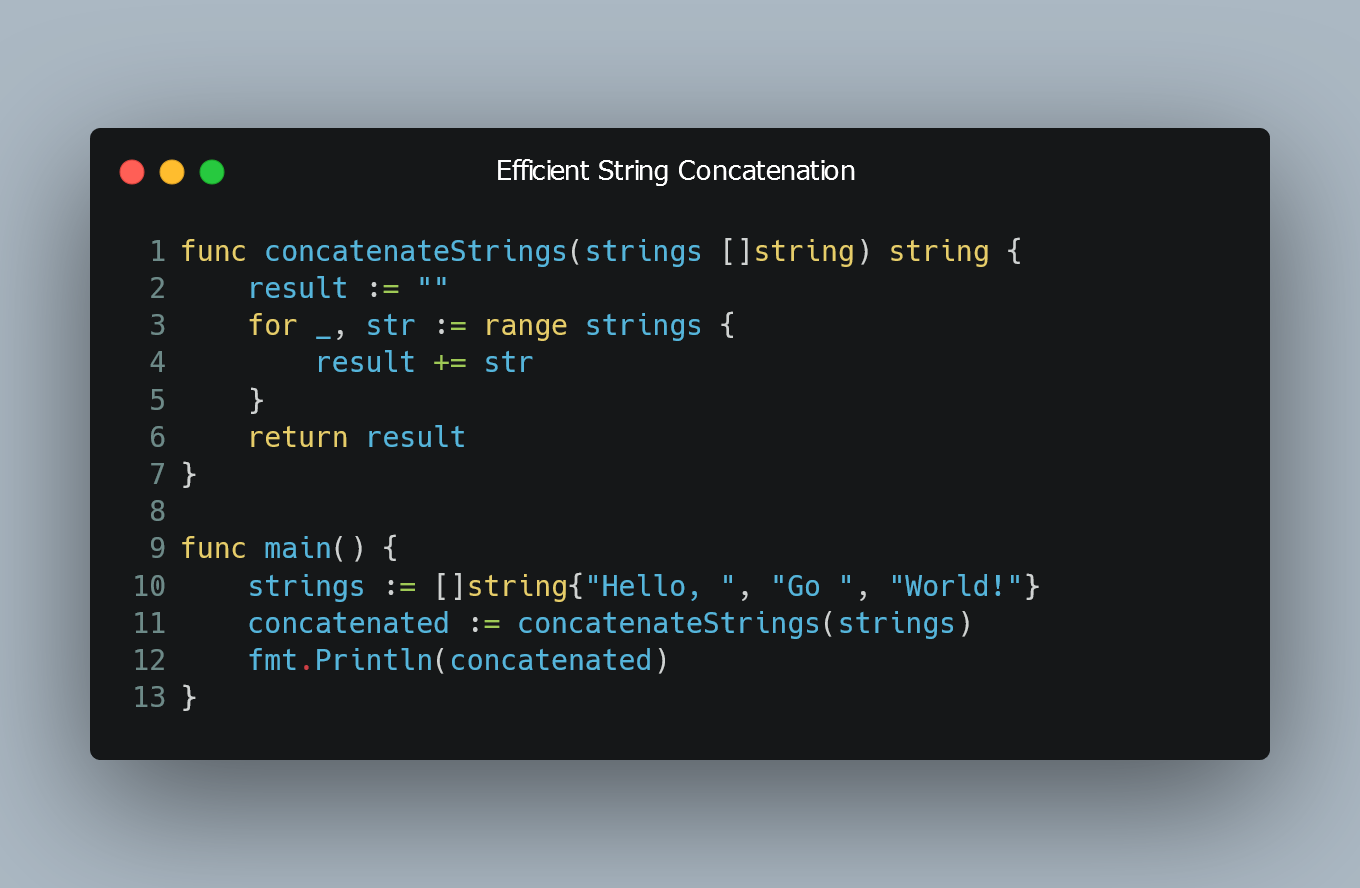
String concatenation is a common operation in programming, and in Go, there are several methods to efficiently concatenate strings.
Using the +
Operator
The simplest way to concatenate strings in Go is by using the +
operator. However, it is not the most efficient method, especially when concatenating multiple strings in a loop. The reason is that strings in Go are immutable, meaning every concatenation creates a new string with the combined value.
Example
func concatenateStrings(strings []string) string {
result := ""
for _, str := range strings {
result += str
}
return result
}
func main() {
strings := []string{"Hello, ", "Go ", "World!"}
concatenated := concatenateStrings(strings)
fmt.Println(concatenated)
}
Using strings.Join()
A more efficient approach to concatenate strings in Go is by using the strings.Join()
function from the strings
package. It takes a slice of strings and a separator as input and efficiently concatenates them into a single string.
Example
import (
"fmt"
"strings"
)
func main() {
strings := []string{"Hello, ", "Go ", "World!"}
concatenated := strings.Join(strings, "")
fmt.Println(concatenated)
}
Using strings.Builder
For the most efficient string concatenation in Go, especially when dealing with large strings or many concatenations, use the strings.Builder
type from the strings
package. strings.Builder
is a mutable type designed to efficiently build strings by reducing unnecessary allocations.
Example
import (
"fmt"
"strings"
)
func main() {
strings := []string{"Hello, ", "Go ", "World!"}
var builder strings.Builder
for _, str := range strings {
builder.WriteString(str)
}
concatenated := builder.String()
fmt.Println(concatenated)
}
By employing these methods, you can efficiently concatenate strings in Go while avoiding unnecessary overhead and allocations, which is especially crucial when dealing with performance-sensitive applications.
0 Comment