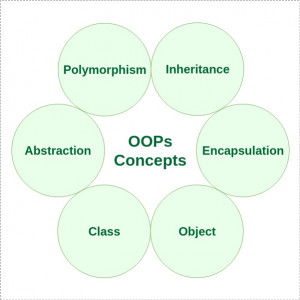
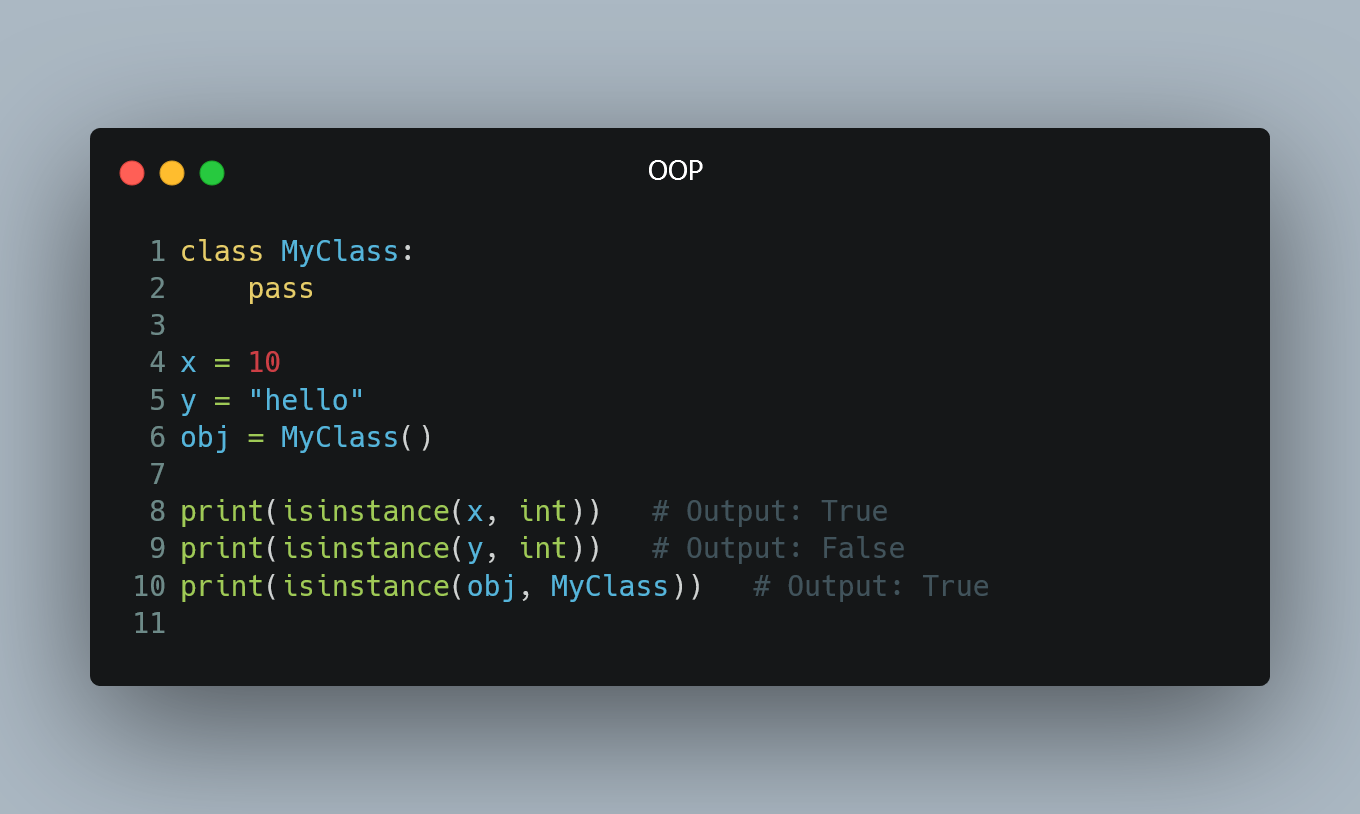
In Python, both type()
and instance()
are used to check the type of an object. However, they have different functionalities and use cases.
type()
The type()
function is used to determine the type of an object. It returns the type as a class object.
Example:
x = 10
y = "hello"
print(type(x)) # Output: <class 'int'>
print(type(y)) # Output: <class 'str'>
In this example, we use type()
to check the types of variables x
and y
, which are an integer and a string, respectively.
isinstance()
The instance()
function is used to check whether an object is an instance of a specified class or its subclasses. It returns True
if the object is an instance of the class, otherwise False
.
Example:
class MyClass:
pass
x = 10
y = "hello"
obj = MyClass()
print(isinstance(x, int)) # Output: True
print(isinstance(y, int)) # Output: False
print(isinstance(obj, MyClass)) # Output: True
In this example, we use isinstance()
to check if x
is an instance of the int
class, if y
is an instance of the int
class (which it is not), and if obj
is an instance of the MyClass
class.
Key Differences
-
Functionality:
type()
returns the actual type of an object, represented as a class object, whereasisinstance()
checks if an object is an instance of a specified class or its subclasses, returningTrue
orFalse
accordingly. -
Inheritance:
type()
does not consider inheritance. For example, for a subclass,type()
returns the subclass type, not the parent class type.isinstance()
considers inheritance and returnsTrue
for both the class and its subclasses. -
Usage: Use
type()
when you need to know the exact type of an object, and useisinstance()
when you want to check if an object belongs to a specific class or its subclasses.
0 Comment