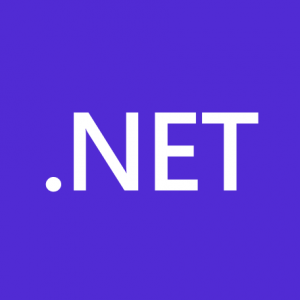
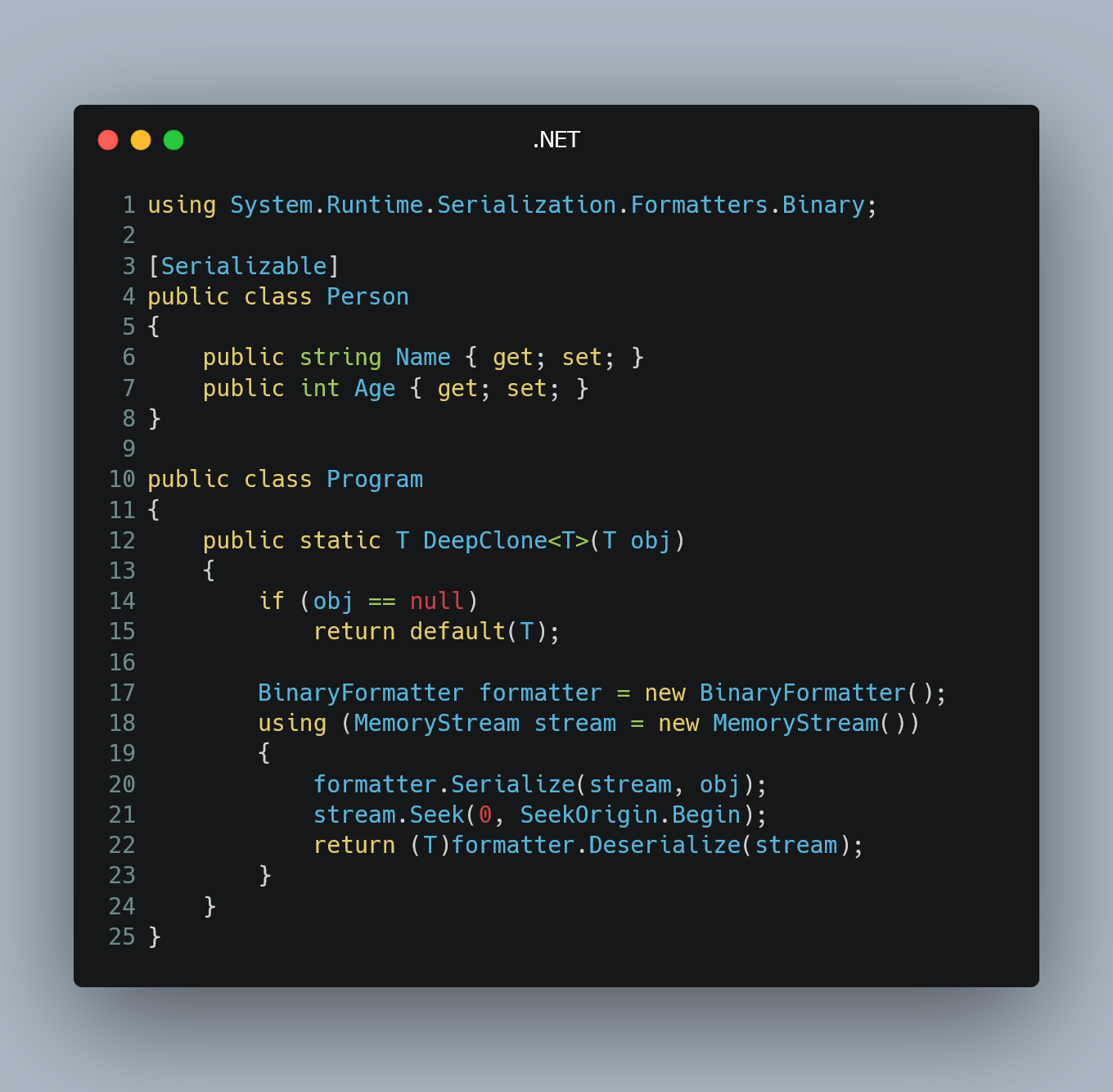
Deep cloning an object involves creating an entirely new copy of the object and all its nested objects, rather than copying references to the original object's properties.
Using the BinaryFormatter Class (Binary Serialization)
C# provides the BinaryFormatter
class, which can be used to perform deep cloning through binary serialization.
Example:
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[Serializable]
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Program
{
public static T DeepClone<T>(T obj)
{
if (obj == null)
return default(T);
BinaryFormatter formatter = new BinaryFormatter();
using (MemoryStream stream = new MemoryStream())
{
formatter.Serialize(stream, obj);
stream.Seek(0, SeekOrigin.Begin);
return (T)formatter.Deserialize(stream);
}
}
public static void Main()
{
Person originalPerson = new Person { Name = "John", Age = 30 };
Person clonedPerson = DeepClone(originalPerson);
clonedPerson.Name = "Jane";
clonedPerson.Age = 25;
Console.WriteLine($"Original Person: {originalPerson.Name}, {originalPerson.Age}");
Console.WriteLine($"Cloned Person: {clonedPerson.Name}, {clonedPerson.Age}");
}
}
In the above example, we create a Person
class and implement deep cloning using the DeepClone
method. The BinaryFormatter
is used for serialization and deserialization, and the MemoryStream
handles the binary data.
When running the program, you'll see that changes to the cloned object don't affect the original object, demonstrating a successful deep clone.
0 Comment