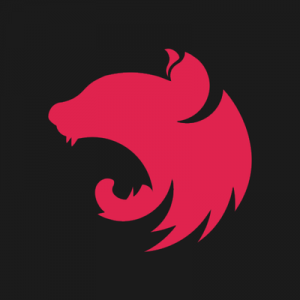
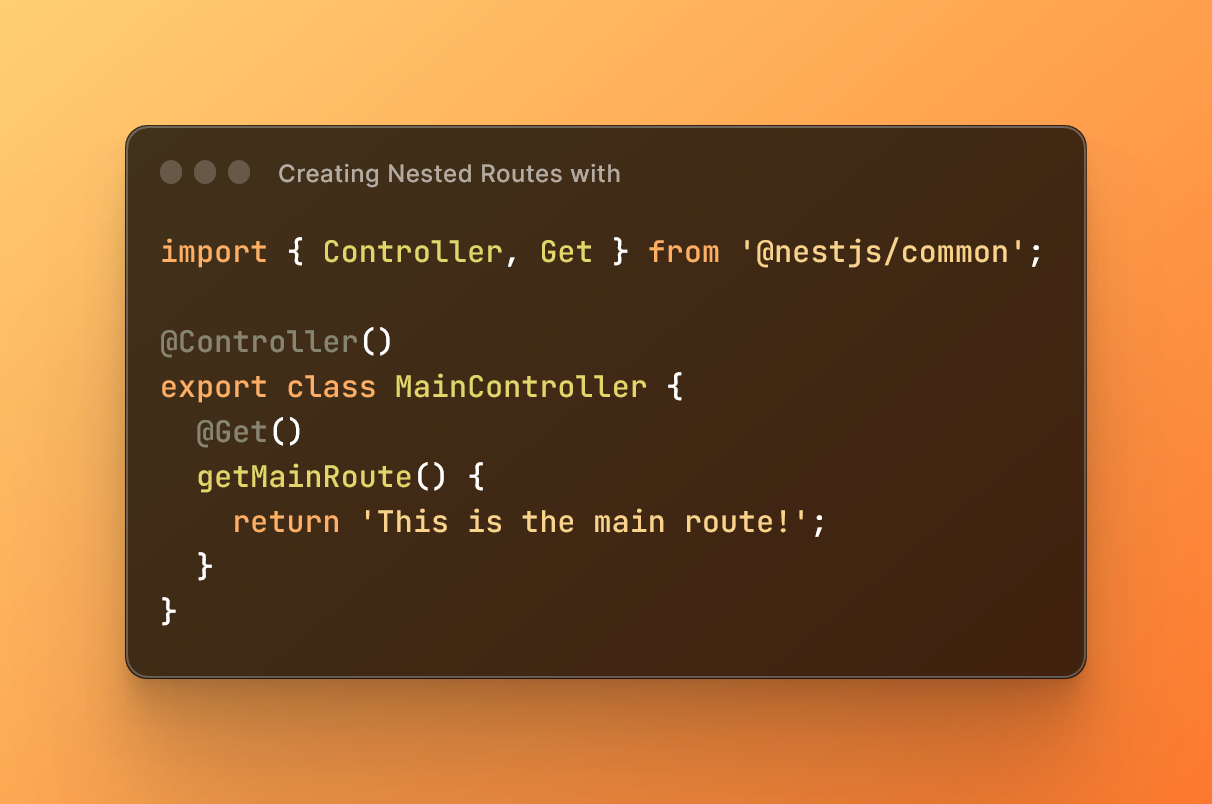
In NestJS, you can create nested routes with parameters to organize your API endpoints and handle more complex data structures. Nested routes allow you to represent hierarchical relationships in your API and pass parameters to different levels of the route.
Setting Up the Main Controller
First, create a main controller that will handle the top-level route. This controller will be responsible for managing the nested routes.
// main.controller.ts
import { Controller, Get } from '@nestjs/common';
@Controller()
export class MainController {
@Get()
getMainRoute() {
return 'This is the main route!';
}
}
In this example, we create a MainController
with a single Get()
endpoint that represents the top-level route.
Creating the Nested Controller
Next, create the nested controller that will handle the nested route.
// nested.controller.ts
import { Controller, Get, Param } from '@nestjs/common';
@Controller('main/:mainId/nested')
export class NestedController {
@Get()
getNestedRoute(@Param('mainId') mainId: string) {
return `This is the nested route for Main ID: ${mainId}!`;
}
}
In this example, we create a NestedController
with a single Get()
endpoint that represents the nested route. We use the @Controller('main/:mainId/nested')
decorator to define the nested route, where :mainId
is the parameter that we will pass to the nested route.
Adding the Nested Controller to the Main Module
Now, add the NestedController
to the main module so that it can be registered with the application.
// app.module.ts
import { Module } from '@nestjs/common';
import { MainController } from './main.controller';
import { NestedController } from './nested.controller';
@Module({
imports: [],
controllers: [MainController, NestedController],
providers: [],
})
export class AppModule {}
In this example, we import the MainController
and NestedController
and add them to the controllers
array of the main module.
Accessing the Nested Route
Now, when you make a request to the nested route with a parameter, it will be handled by the NestedController
.
For example, if you make a GET request to /main/123/nested
, it will be handled by the NestedController
with the mainId
parameter set to 123
. The response will be:
This is the nested route for Main ID: 123!
0 Comment