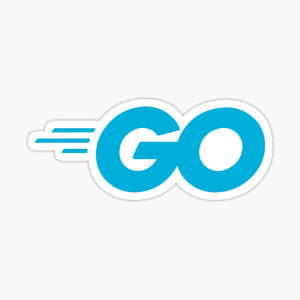
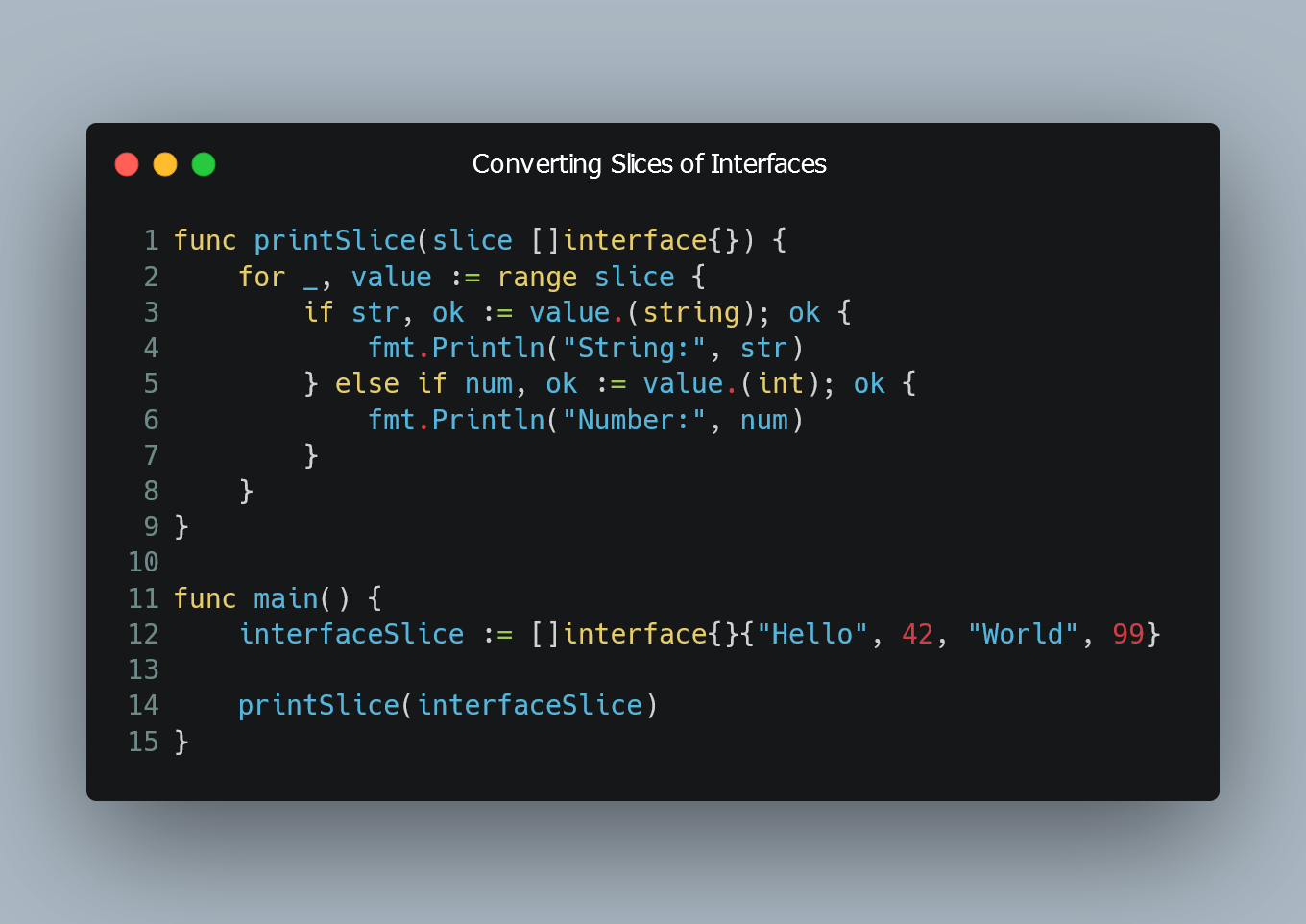
Type converting slices of interfaces in Go allows us to work with specific types contained within an interface slice.
Interface Slice and Concrete Types
In Go, an interface is a type that defines a set of methods. An interface slice, []interface{}
, can hold values of any type that implements the interface. However, to perform operations specific to a particular type, we need to convert the elements of the interface slice back to their concrete types.
Type Assertion for Conversion
Type assertions are used in Go to convert interface values to their underlying concrete types. A type assertion has the following syntax:
value, ok := interfaceValue.(Type)
-
value
is the converted value of the concrete type. -
ok
is a boolean that indicates whether the assertion succeeded (true) or not (false). -
interfaceValue
is the value of the interface to be converted. -
Type
is the concrete type we expect.
Example
func printSlice(slice []interface{}) {
for _, value := range slice {
if str, ok := value.(string); ok {
fmt.Println("String:", str)
} else if num, ok := value.(int); ok {
fmt.Println("Number:", num)
}
}
}
func main() {
interfaceSlice := []interface{}{"Hello", 42, "World", 99}
printSlice(interfaceSlice)
}
In this example, the printSlice
function takes an interface slice as input and uses type assertions to print the elements of the slice with their corresponding types.
Converting with Type Switch
Type switches provide another way to convert elements in an interface slice to their concrete types. It allows us to handle multiple type assertions in a more concise and organized manner.
Example
func printSlice(slice []interface{}) {
for _, value := range slice {
switch v := value.(type) {
case string:
fmt.Println("String:", v)
case int:
fmt.Println("Number:", v)
}
}
}
func main() {
interfaceSlice := []interface{}{"Hello", 42, "World", 99}
printSlice(interfaceSlice)
}
The output will be the same as in the previous example, but this time we use a type switch for the conversion.
Type converting slices of interfaces is an essential skill in Go when dealing with generic containers or interfaces that can hold various types.
0 Comment