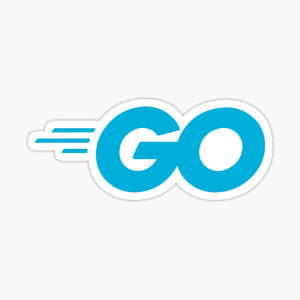
Published in
GO
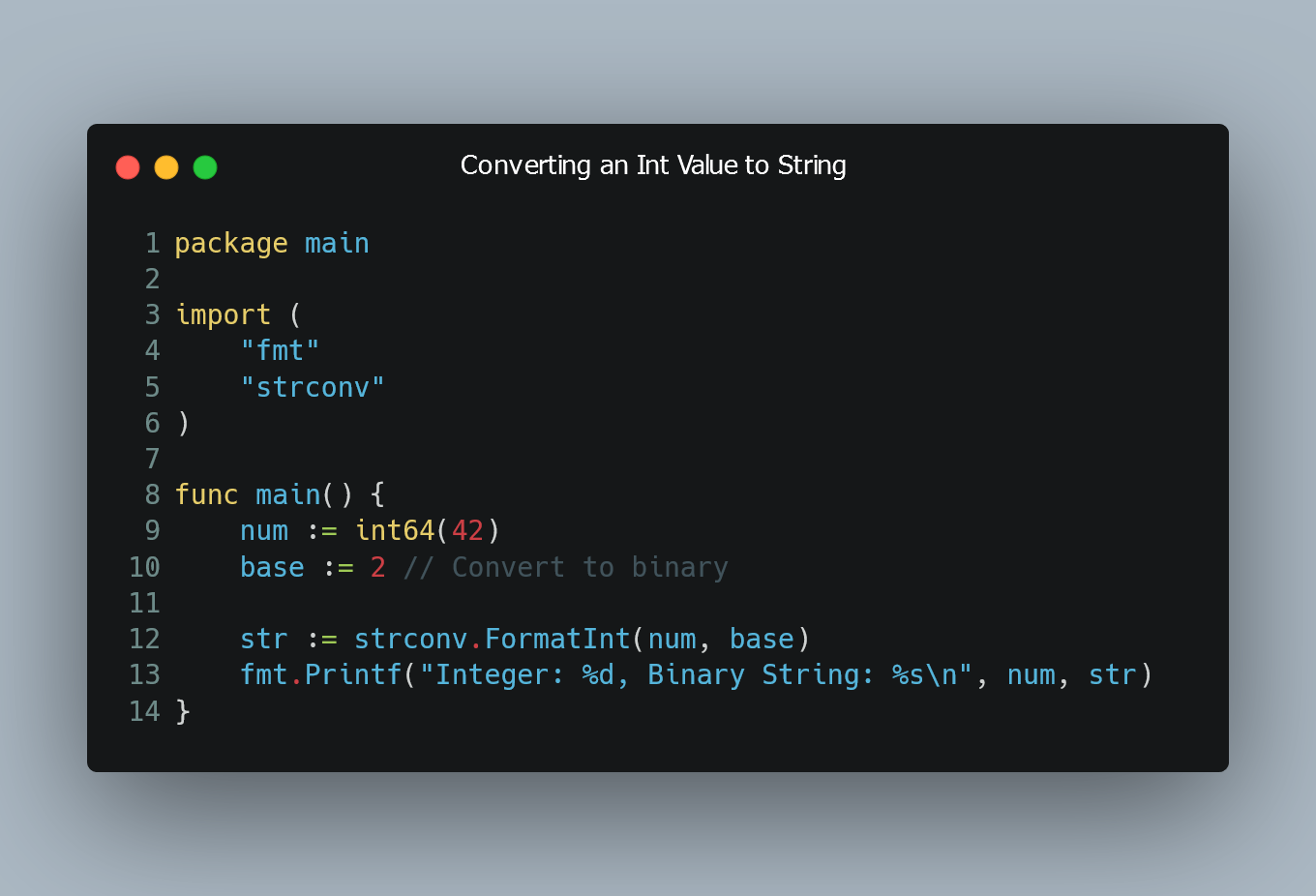
To convert an integer value to a string in Go, you can use the strconv
package, which provides functions for handling string conversions.
Using strconv.Itoa()
The strconv.Itoa()
function is specifically designed to convert an int to its string representation.
Example
package main
import (
"fmt"
"strconv"
)
func main() {
num := 42
str := strconv.Itoa(num)
fmt.Printf("Integer: %d, String: %s\n", num, str)
}
Using fmt.Sprintf()
Another method to convert an int to a string is by using fmt.Sprintf()
.
Example
package main
import "fmt"
func main() {
num := 42
str := fmt.Sprintf("%d", num)
fmt.Printf("Integer: %d, String: %s\n", num, str)
}
Using strconv.FormatInt()
If you need more control over the string conversion, such as specifying the base (e.g., binary, hexadecimal), you can use strconv.FormatInt()
.
Example
package main
import (
"fmt"
"strconv"
)
func main() {
num := int64(42)
base := 2 // Convert to binary
str := strconv.FormatInt(num, base)
fmt.Printf("Integer: %d, Binary String: %s\n", num, str)
}
2 Comments
Can I get to know you
Hello