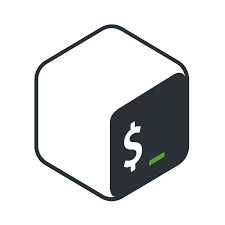
Published in
Bash
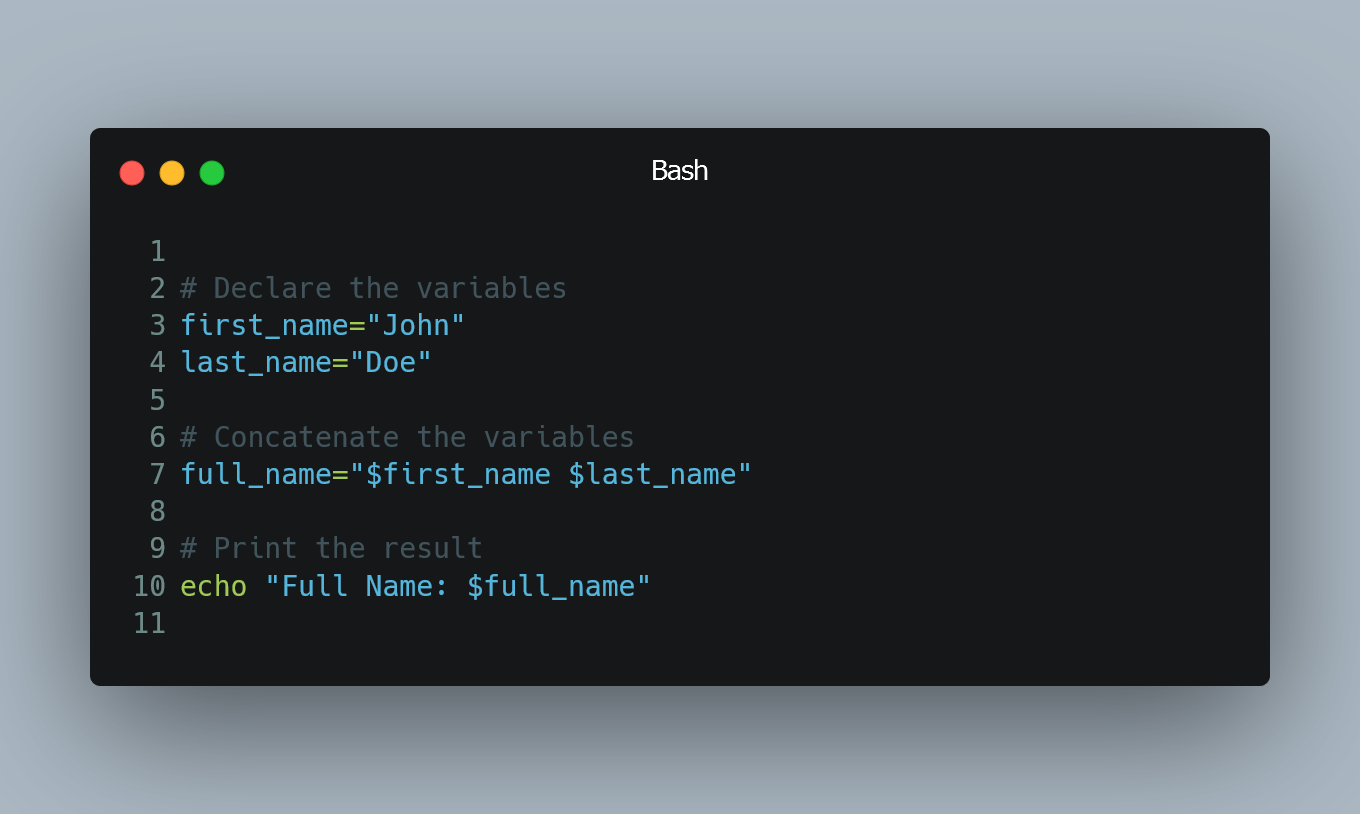
To concatenate string variables in Bash, you can use different methods to combine their values into a single string.
Using the +
operator
You can concatenate string variables using the +
operator.
# Declare the variables
first_name="John"
last_name="Doe"
# Concatenate the variables
full_name="$first_name $last_name"
# Print the result
echo "Full Name: $full_name"
Using the ${}
syntax
You can also use the ${}
syntax to concatenate string variables.
# Declare the variables
fruit="apple"
color="red"
# Concatenate the variables
description="${color} ${fruit}"
# Print the result
echo "Description: $description"
Using string interpolation
String interpolation allows you to combine variables directly within a string.
# Declare the variables
animal="cat"
sound="meow"
# Concatenate the variables within a string
echo "The $animal makes a $sound sound."
Using +=
operator
The +=
operator appends the value of one variable to another.
# Declare the variables
greeting="Hello"
name="Alice"
# Concatenate the variables
greeting+=" $name"
# Print the result
echo "$greeting"
Using printf
command
The printf
command can be used to format and concatenate strings.
# Declare the variables
item="apple"
quantity=5
# Concatenate the variables using printf
output=$(printf "I have %d %ss." $quantity $item)
# Print the result
echo "$output"
Using echo
command with multiple arguments
You can also use multiple arguments with the echo
command to concatenate strings.
# Declare the variables
language="Bash"
version="5"
# Concatenate the variables using echo
echo "Using" $language "version" $version
0 Comment