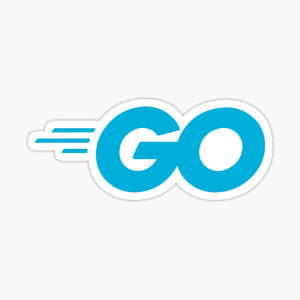
In Go, you can concatenate two slices to create a new slice that contains elements from both original slices.
Using Append
The append()
function in Go is a flexible way to concatenate slices. It takes the destination slice followed by the elements to be appended and returns a new slice with the concatenated elements.
Example
package main
import "fmt"
func main() {
slice1 := []int{1, 2, 3}
slice2 := []int{4, 5, 6}
concatenated := append(slice1, slice2...)
fmt.Println(concatenated)
}
In this example, slice1
and slice2
are concatenated using the append()
function with ...
to unpack the elements of slice2
into individual arguments.
Using a Loop
If you want to concatenate slices without modifying the original slices, you can use a loop to copy the elements into a new slice.
Example
package main
import "fmt"
func main() {
slice1 := []string{"apple", "banana", "orange"}
slice2 := []string{"grape", "kiwi"}
concatenated := make([]string, len(slice1)+len(slice2))
copy(concatenated, slice1)
copy(concatenated[len(slice1):], slice2)
fmt.Println(concatenated)
}
In this example, a new slice concatenated
is created with enough capacity to hold all elements from both slice1
and slice2
. The copy()
function is then used to copy the elements from both slices into the new concatenated
slice.
0 Comment