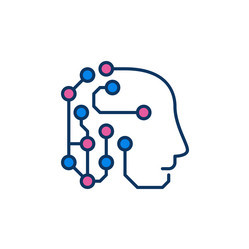
When working with classes in programming languages like C#, Java, or Python, it's essential to override the GetHashCode
method to provide a meaningful and efficient implementation for hashing objects. A good GetHashCode
implementation is crucial for optimal performance in hash-based collections like dictionaries and hash sets.
Understanding GetHashCode
What is GetHashCode?
In many programming languages, including C#, Java, and Python, the GetHashCode
method is used to generate a hash code for an object. Hash codes are used by hash-based collections to quickly index and retrieve elements. An ideal GetHashCode
method should provide a unique hash code for each distinct object while distributing the hash codes evenly across the possible range.
Why Override GetHashCode?
By default, the base implementation of GetHashCode
provided by the Object class is based on the memory address of the object. However, this default implementation might not produce optimal results for custom classes, especially when you want to use instances of the class as keys in hash-based collections.
Selecting the Best Algorithm
1. Consider Object Equality
The primary rule for a good GetHashCode
implementation is that objects that are equal (according to their Equals
method) should have the same hash code. This is essential for maintaining the integrity of hash-based collections.
2. Avoid Collisions
A collision occurs when two distinct objects produce the same hash code. While collisions are inevitable due to the finite range of hash codes, a good algorithm should aim to minimize their occurrence to prevent performance degradation in hash-based collections.
3. Distribute Hash Codes Evenly
A well-designed GetHashCode
algorithm should distribute hash codes as evenly as possible across the entire range of possible hash values. This ensures that hash-based collections can efficiently distribute elements in their internal data structures, providing faster access times.
4. Combine Multiple Fields
For classes with multiple fields, combine the hash codes of those fields to create a composite hash code. This helps create a more unique hash code for each object, considering all relevant attributes.
5. Use Prime Numbers
Multiplying hash codes by prime numbers can help improve the distribution of hash codes and reduce the likelihood of collisions. Common choices for prime numbers are 31 or 37.
6. XOR for Combining
When combining multiple hash codes, use bitwise XOR (^) operation instead of addition or multiplication. XOR helps ensure that the order of fields in the combination doesn't affect the resulting hash code.
7. Consider Immutable Fields
If the class contains immutable fields, you can often cache the hash code once it's computed since immutable objects' hash codes won't change.
Example (C#)
public override int GetHashCode()
{
unchecked
{
int hash = 17;
hash = hash * 31 + field1.GetHashCode();
hash = hash * 31 + field2.GetHashCode();
// ... add more fields if needed
return hash;
}
}
In this example, we override GetHashCode
for a class with two fields, field1
and field2
. We combine their hash codes using the XOR operator and multiply by the prime number 31 for improved distribution.
0 Comment