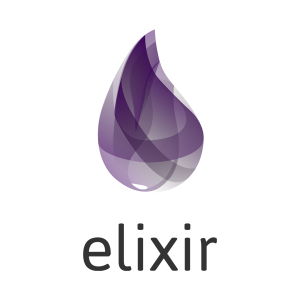
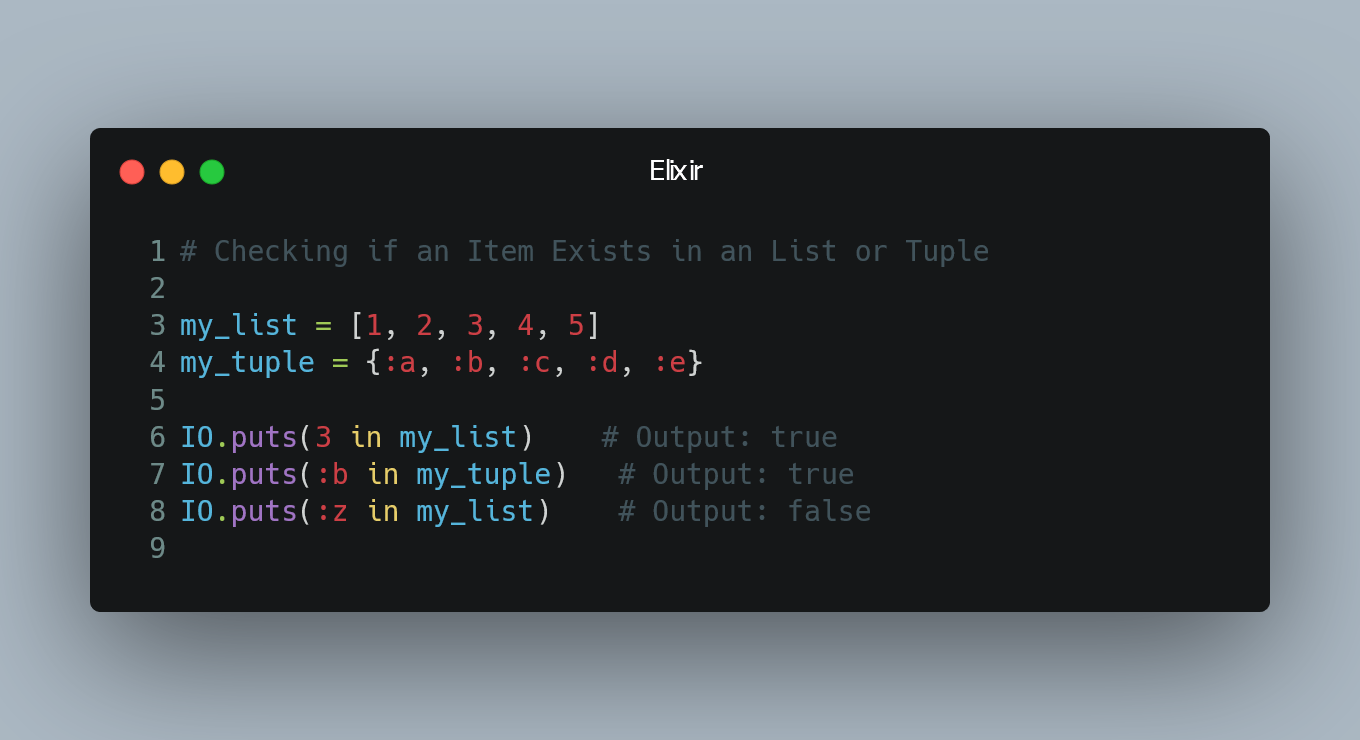
In Elixir, you can determine if an item exists in a list or tuple using various built-in functions. Checking for the presence of an item is a common operation when working with collections in Elixir.
Using the in Operator
The in
operator is a concise way to check if an item is present in a list or tuple.
Example:
my_list = [1, 2, 3, 4, 5]
my_tuple = {:a, :b, :c, :d, :e}
IO.puts(3 in my_list) # Output: true
IO.puts(:b in my_tuple) # Output: true
IO.puts(:z in my_list) # Output: false
In this example, we use the in
operator to check if the item 3
exists in the list my_list
and if the atom :b
exists in the tuple my_tuple
. We also check for an item that does not exist in the list to demonstrate a false
result.
Using Enum.member?/2
The Enum.member?/2
function allows you to explicitly check if an item is a member of a list or tuple.
Example:
my_list = [10, 20, 30]
my_tuple = {:x, :y, :z}
IO.puts(Enum.member?(my_list, 20)) # Output: true
IO.puts(Enum.member?(my_tuple, :y)) # Output: true
IO.puts(Enum.member?(my_list, 40)) # Output: false
In this example, we use Enum.member?/2
to check if 20
exists in my_list
, :y
exists in my_tuple
, and 40
exists in my_list
.
Using Kernel.elem/2
The Kernel.elem/2
function is another way to check if an item is present in a tuple.
Example:
my_tuple = {:apple, :banana, :orange}
IO.puts(Kernel.elem(my_tuple, :banana)) # Output: true
IO.puts(Kernel.elem(my_tuple, :grape)) # Output: false
In this example, we use Kernel.elem/2
to check if :banana
exists in my_tuple
and if :grape
exists in my_tuple
.
0 Comment