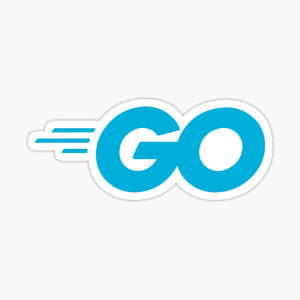
To determine whether a map contains a specific key in Go, we can use the built-in map
functionality.
Using the Comma Ok Idiom
In Go, the comma ok idiom is a common and efficient way to check if a map contains a key. It utilizes the second return value of a map lookup to indicate whether the key exists in the map or not.
Example
func main() {
// Create a map
myMap := map[string]int{
"apple": 1,
"banana": 2,
"orange": 3,
}
// Check if a key exists
key := "banana"
if value, ok := myMap[key]; ok {
fmt.Println(key, "exists in the map with value:", value)
} else {
fmt.Println(key, "does not exist in the map.")
}
}
Checking Without the Value
If you only want to check for the existence of a key and do not require its corresponding value, you can simply ignore the value in the comma ok idiom.
Example
func main() {
myMap := map[string]int{
"apple": 1,
"banana": 2,
"orange": 3,
}
key := "orange"
if _, ok := myMap[key]; ok {
fmt.Println(key, "exists in the map.")
} else {
fmt.Println(key, "does not exist in the map.")
}
}
Using a Helper Function
If you find yourself frequently checking for key existence in maps, you can create a helper function to encapsulate the check and make your code more readable.
Example
func keyExists(myMap map[string]int, key string) bool {
_, ok := myMap[key]
return ok
}
func main() {
myMap := map[string]int{
"apple": 1,
"banana": 2,
"orange": 3,
}
key := "apple"
if keyExists(myMap, key) {
fmt.Println(key, "exists in the map.")
} else {
fmt.Println(key, "does not exist in the map.")
}
}
By following these methods, you can easily check if a map contains a key in Go and take appropriate actions based on the result.
0 Comment