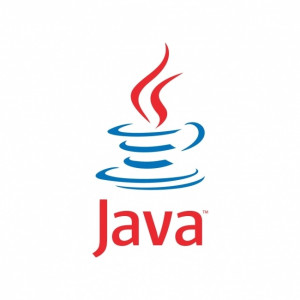
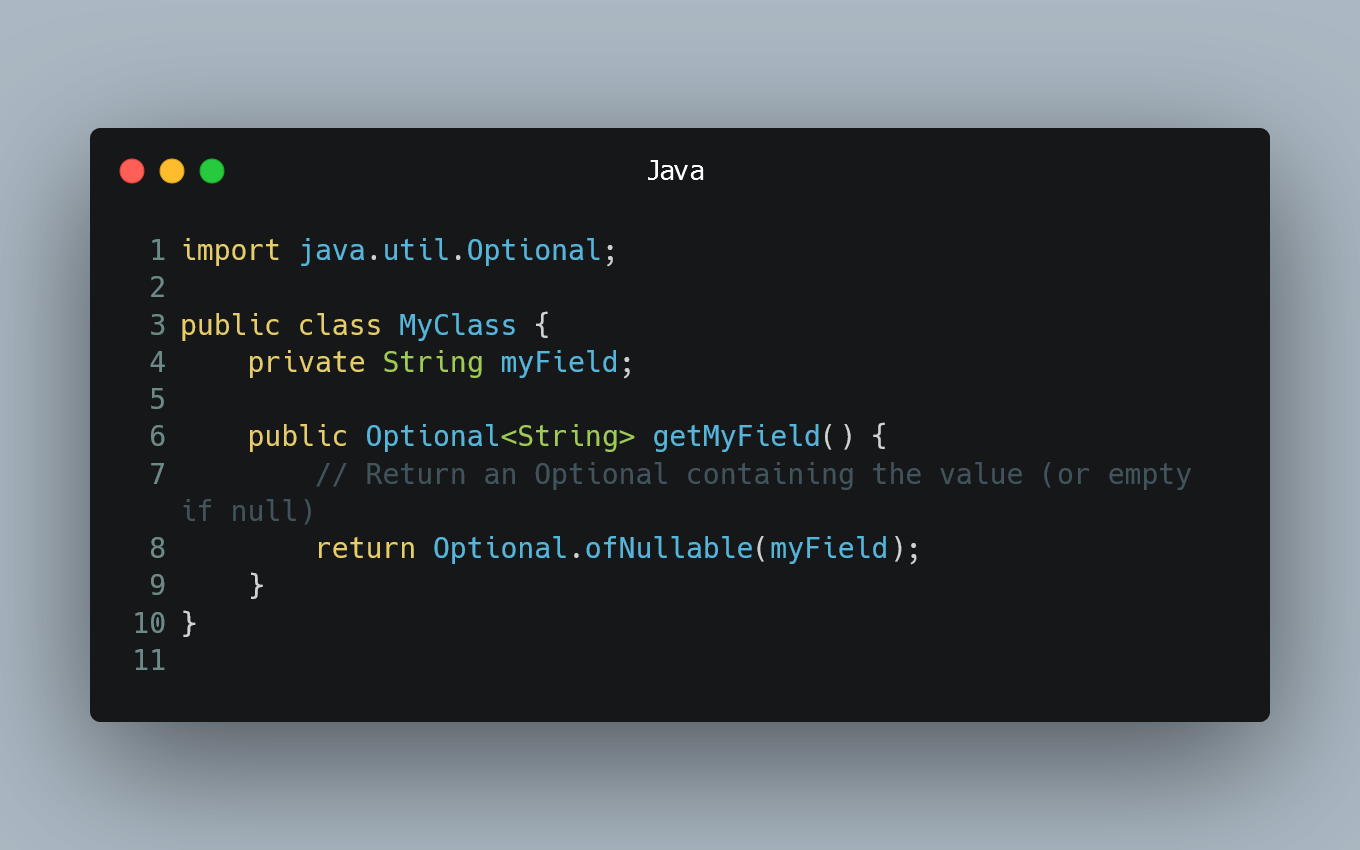
Null checks in Java can lead to messy and error-prone code. Fortunately, there are techniques and best practices you can follow to minimize null checks and improve the reliability of your code.
Use Objects.requireNonNull()
Java provides the Objects.requireNonNull()
method to handle null values more efficiently. Instead of manually checking for nulls, you can use this method to validate the input and throw a NullPointerException
with a specified message if the value is null.
Example:
import java.util.Objects;
public class MyClass {
private String myField;
public MyClass(String value) {
myField = Objects.requireNonNull(value, "value cannot be null");
}
}
In this example, we use Objects.requireNonNull()
to validate the value
parameter in the constructor of MyClass
. If the value
is null, a NullPointerException
will be thrown with the specified message.
Use Optional
Java 8 introduced the Optional
class to handle situations where a value might be absent. Instead of returning null, you can return an Optional
object, which either contains the value or indicates its absence.
Example:
import java.util.Optional;
public class MyClass {
private String myField;
public Optional<String> getMyField() {
// Return an Optional containing the value (or empty if null)
return Optional.ofNullable(myField);
}
}
In this example, the getMyField()
method returns an Optional
object that contains the value of myField
, if not null. Otherwise, it returns an empty Optional
.
Use Default Values with Optional
You can combine Optional
with default values to handle null values more elegantly. The orElse()
method returns the value if it's present or the specified default value if it's absent.
Example:
import java.util.Optional;
public class MyClass {
private String myField;
public String getMyField() {
return Optional.ofNullable(myField).orElse("default value");
}
}
In this example, getMyField()
returns the value of myField
if it's not null, or "default value" if it's null.
0 Comment